adamralph / Bullseye

Bullseye
Bullseye is a .NET library that runs a target dependency graph.
Bullseye targets can do anything. They are not restricted to building .NET projects.
Platform support: .NET Standard 2.0 and upwards.
Quick start
-
Create a .NET console app named
targets
and add a reference to Bullseye. -
Replace the contents of
Program.cs
with:using static Bullseye.Targets; class Program { static void Main(string[] args) { Target("default", () => System.Console.WriteLine("Hello, world!")); RunTargetsAndExit(args); } }
-
Run the app. E.g.
dotnet run
or F5 in Visual Studio:
Voilà ! You've just written and run your first Bullseye program. You will see output similar to:
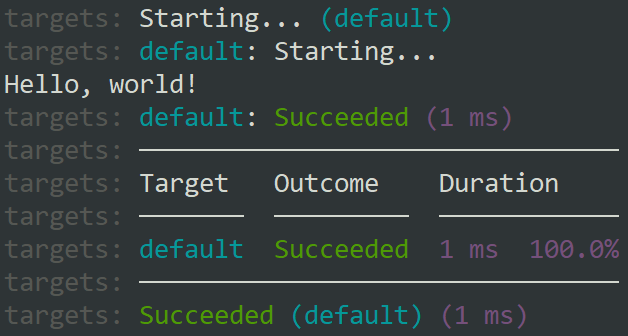
For help, run dotnet run -- --help
.
Also see the async quick start.
Defining dependencies
Target("make-tea", () => Console.WriteLine("Tea made."));
Target("drink-tea", DependsOn("make-tea"), () => Console.WriteLine("Ahh... lovely!"));
Target("walk-dog", () => Console.WriteLine("Walkies!"));
Target("default", DependsOn("drink-tea", "walk-dog"));
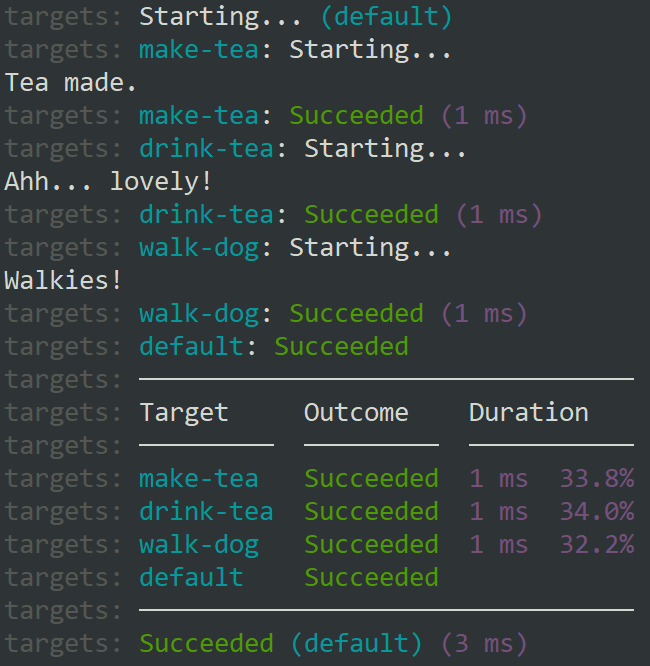
Enumerable inputs
Target(
"eat-biscuits",
ForEach("digestives", "chocolate hobnobs"),
biscuits => Console.WriteLine($"Mmm...{biscuits}! Nom nom."));
dotnet run -- eat-biscuits
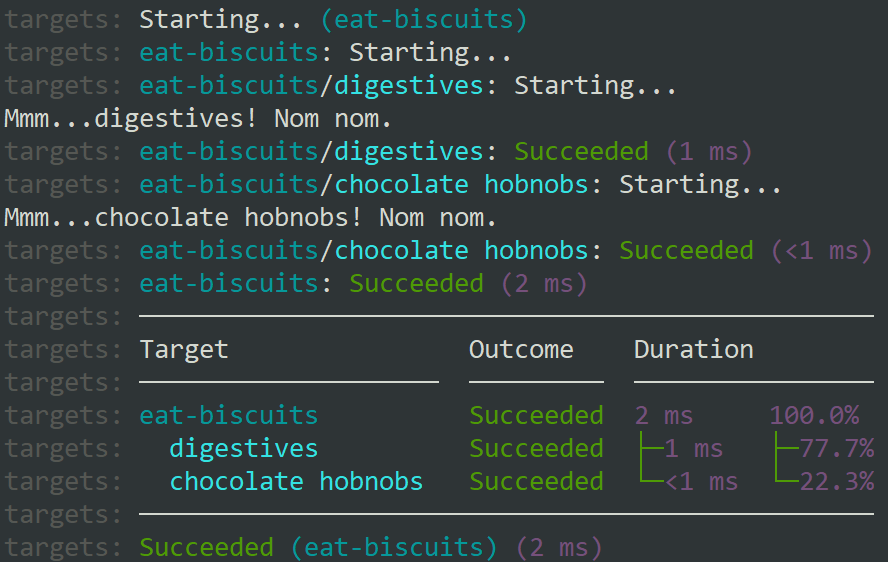
Sample wrapper scripts
-
build.cmd
@echo Off dotnet run --project targets -- %*
-
build.sh
#!/usr/bin/env bash set -euo pipefail dotnet run --project targets -- "[email protected]"
-
build.ps1
$ErrorActionPreference = "Stop"; dotnet run --project targets -- $args
Command line arguments
Generally, all the command line arguments passed to Program.cs
should be passed along to Bullseye, as shown in the quick start above (RunTargetsAndExit(args);
). This is because Bullseye effectively provides a command line interface, with options for displaying a list of targets, performing dry runs, suppressing colour, and more. For full details of the command line options, run your targets project supplying the --help
(-h
/-?
) option:
dotnet run --project targets -- --help
./build.cmd --help
./build.sh -h
./build.ps1 -?
You can also handle custom arguments in Program.cs
, but you should ensure that only valid arguments are passed along to Bullseye and that the help text contains both your custom arguments and the arguments supported by Bullseye. A good way to do this is to use a command line parsing package to define your custom arguments, and to provide translation between the package and Bullseye. For example, see the test projects for:
Non-static API
For most cases, the static API described above is sufficient. For more complex scenarios where a number of target collections are required, the non-static API may be used.
var targets1 = new Targets();
targets1.Add("foo", () => Console.Out.WriteLine("foo1"));
var targets2 = new Targets();
targets2.Add("foo", () => Console.Out.WriteLine("foo2"));
targets1.RunWithoutExiting(args);
targets2.RunWithoutExiting(args);
NO_COLOR
Bullseye supports NO_COLOR.
FAQ
Can I force a pause before exiting when debugging in Visual Studio 2017 (or earlier)?
Yes! Add the following line anywhere before calling RunTargetsAndExit
/RunTargetsAndExitAsync
:
AppDomain.CurrentDomain.ProcessExit += (s, e) => Console.ReadKey();
Note that the common way to do this for .NET console apps is to add a line such as the following before the end of the Program.Main
method:
Console.ReadKey();
This does not work after calling RunTargetsAndExit
/RunTargetsAndExit
because that is the final statement that will be executed.
In Visual Studio 2019 and later, .NET console apps pause before exiting by default, so none of this is required.
Who's using Bullseye?
To name a few:
- AspNetCore.AsyncInitialization
- Config.SqlStreamStore
- ConfigR
- Elastic
- EssentialMVVM
- FakeItEasy
- HumanBytes
- Ibento
- IdentityModel
- IdentityServer
- Iso8601DurationHelper
- Linq.Extras
- LittleForker
- LykkeOSS
- Marten
- MinVer
- Particular
- ProxyKit
- PseudoLocalizer
- Radical Framework
- RealWorld
- SelfInitializingFakes
- SendComics
- SqlStreamStore.Locking
- SQLStreamStore
- Statik
- Tasty
- TemplatedConfiguration
- xBehave.net
- Xenial
Feel free to send a pull request to add your repo or organisation to this list!
Target by Franck Juncker from the Noun Project.