z7zmey / Php Parser
Licence: mit
PHP parser written in Go
Stars: ✭ 787
Programming Languages
go
31211 projects - #10 most used programming language
Projects that are alternatives of or similar to Php Parser
Exprtk
C++ Mathematical Expression Parsing And Evaluation Library
Stars: ✭ 301 (-61.75%)
Mutual labels: ast, parser
Esprima
ECMAScript parsing infrastructure for multipurpose analysis
Stars: ✭ 6,391 (+712.07%)
Mutual labels: ast, parser
Astroid
A common base representation of python source code for pylint and other projects
Stars: ✭ 310 (-60.61%)
Mutual labels: ast, parser
Tolerant Php Parser
An early-stage PHP parser designed for IDE usage scenarios.
Stars: ✭ 717 (-8.89%)
Mutual labels: ast, parser
Tiny Compiler
A tiny compiler for a language featuring LL(2) with Lexer, Parser, ASM-like codegen and VM. Complex enough to give you a flavour of how the "real" thing works whilst not being a mere toy example
Stars: ✭ 425 (-46%)
Mutual labels: ast, parser
Jsqlparser
JSqlParser parses an SQL statement and translate it into a hierarchy of Java classes. The generated hierarchy can be navigated using the Visitor Pattern
Stars: ✭ 3,405 (+332.66%)
Mutual labels: ast, parser
Javaparser
Java 1-15 Parser and Abstract Syntax Tree for Java, including preview features to Java 13
Stars: ✭ 3,972 (+404.7%)
Mutual labels: ast, parser
Escaya
An blazing fast 100% spec compliant, incremental javascript parser written in Typescript
Stars: ✭ 217 (-72.43%)
Mutual labels: ast, parser
Php Parser
🌿 NodeJS PHP Parser - extract AST or tokens (PHP5 and PHP7)
Stars: ✭ 400 (-49.17%)
Mutual labels: ast, parser
Cppast.net
CppAst is a .NET library providing a C/C++ parser for header files powered by Clang/libclang with access to the full AST, comments and macros
Stars: ✭ 228 (-71.03%)
Mutual labels: ast, parser
Meriyah
A 100% compliant, self-hosted javascript parser - https://meriyah.github.io/meriyah
Stars: ✭ 690 (-12.33%)
Mutual labels: ast, parser
Bblfshd
A self-hosted server for source code parsing
Stars: ✭ 297 (-62.26%)
Mutual labels: ast, parser
Vermin
Concurrently detect the minimum Python versions needed to run code
Stars: ✭ 218 (-72.3%)
Mutual labels: ast, parser
Ratel Core
High performance JavaScript to JavaScript compiler with a Rust core
Stars: ✭ 367 (-53.37%)
Mutual labels: ast, parser
Tatsu
竜 TatSu generates Python parsers from grammars in a variation of EBNF
Stars: ✭ 198 (-74.84%)
Mutual labels: ast, parser
Astexplorer
A web tool to explore the ASTs generated by various parsers.
Stars: ✭ 4,330 (+450.19%)
Mutual labels: ast, parser
Tenko
An 100% spec compliant ES2021 JavaScript parser written in JS
Stars: ✭ 490 (-37.74%)
Mutual labels: ast, parser
PHP Parser written in Go
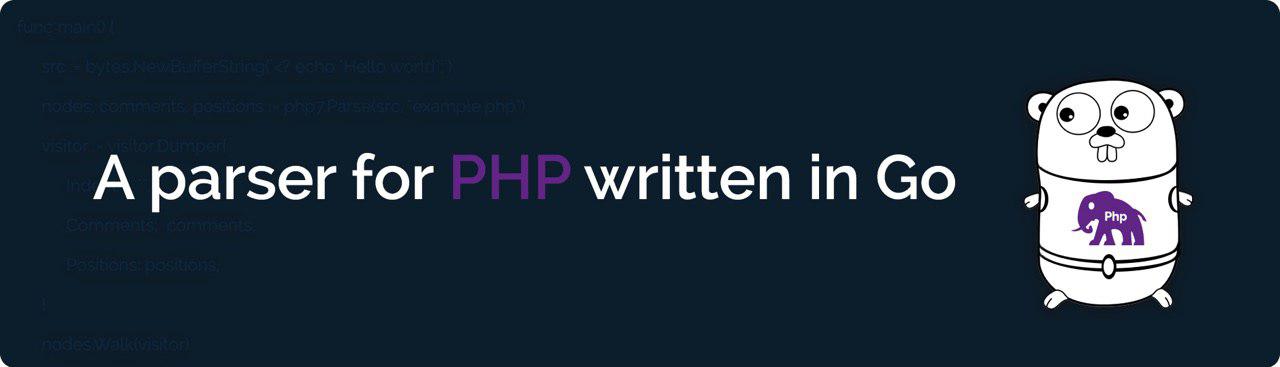
This project uses goyacc and ragel tools to create PHP parser. It parses source code into AST. It can be used to write static analysis, refactoring, metrics, code style formatting tools.
demo
Try it online:Features:
- Fully support PHP 5 and PHP 7 syntax
- Abstract syntax tree (AST) representation
- Traversing AST
- Resolving namespaced names
- Parsing syntax-invalid PHP files
- Saving and printing free-floating comments and whitespaces
Who Uses
VKCOM/noverify - NoVerify is a pretty fast linter for PHP
quasilyte/phpgrep - phpgrep is a tool for syntax-aware PHP code search
Usage example
package main
import (
"log"
"os"
"github.com/z7zmey/php-parser/pkg/cfg"
"github.com/z7zmey/php-parser/pkg/errors"
"github.com/z7zmey/php-parser/pkg/parser"
"github.com/z7zmey/php-parser/pkg/version"
"github.com/z7zmey/php-parser/pkg/visitor/dumper"
)
func main() {
src := []byte(`<? echo "Hello world";`)
// Error handler
var parserErrors []*errors.Error
errorHandler := func(e *errors.Error) {
parserErrors = append(parserErrors, e)
}
// Parse
rootNode, err := parser.Parse(src, cfg.Config{
Version: &version.Version{Major: 5, Minor: 6},
ErrorHandlerFunc: errorHandler,
})
if err != nil {
log.Fatal("Error:" + err.Error())
}
// Dump
goDumper := dumper.NewDumper(os.Stdout).
WithTokens().
WithPositions()
rootNode.Accept(goDumper)
}
Roadmap
- Control Flow Graph (CFG)
- PHP8
Install
go get github.com/z7zmey/php-parser/cmd/php-parser
CLI
php-parser [flags] <path> ...
flag | type | description |
---|---|---|
-p | bool | print filepath |
-e | bool | print errors |
-d | bool | dump in golang format |
-r | bool | resolve names |
-prof | string | start profiler: [cpu, mem, trace] |
-phpver | string | php version (default: 7.4) |
Namespace resolver
Namespace resolver is a visitor that resolves nodes fully qualified name and saves into map[node.Node]string
structure
- For
Class
,Interface
,Trait
,Function
,Constant
nodes it saves name with current namespace. - For
Name
,Relative
,FullyQualified
nodes it resolvesuse
aliases and saves a fully qualified name.
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].