jxnblk / Axs
Programming Languages
Projects that are alternatives of or similar to Axs
Axs
Stupid simple style components for React
If you know React and you know CSS, you already know how to use this.
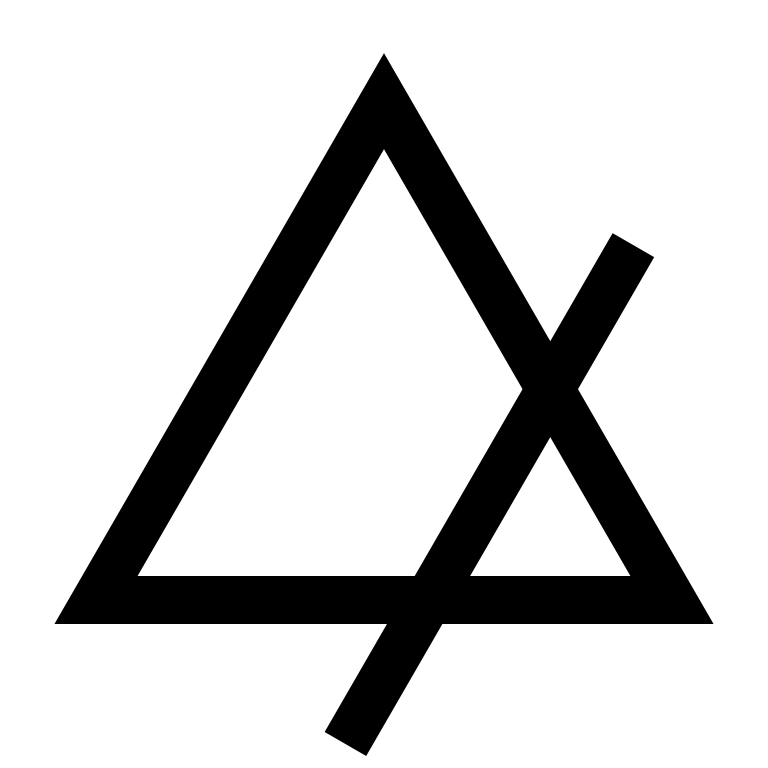
npm i axs
const Heading = props =>
<Base.h2
{...props}
css={`
color: tomato;
`}
/>
A hand axe is a prehistoric stone tool... the longest-used tool in human history.
Features
- Minimal API surface area
- One component, three props
- React component-based API
- Server side rendering with no additional setup
- No custom Babel plugins or additional configuration needed
- Works in iframes
- 0.7 kb in under 100 LOC
Usage
Basic
Create components by wrapping the Base component and passing props and a css
prop to add style.
const Heading = props =>
<Base
{...props}
css={`
color: tomato;
`}
/>
CSS syntax
The css
prop uses stylis for a CSS-like syntax that allows pseudo-classes, media queries, animations, and nested selectors to be defined inline.
See the stylis docs for more info.
const Heading = props =>
<Base
{...props}
css={`
color: tomato;
&:hover {
color: black;
}
`}
/>
HTML elements
To change the underlying HTML element, pass a tag name to the is
prop.
const Heading = props =>
<Base
is='h1'
{...props}
css={`
color: tomato;
`}
/>
Alternatively, the Base component is decorated with keys for all valid HTML elements, which map to the is
prop.
const Heading = props =>
<Base.h1
{...props}
css={`
color: tomato;
`}
/>
When using the component, the underlying element can be changed on a per-instance basis using the is
prop.
This is especially helpful for ensuring the use of correct HTML semantics, while keeping the component styles decoupled.
<Heading is='h2'>
Hello
</Heading>
Dynamic styles
Styles can be set dynamically based on props.
const Heading = props =>
<Base.h2
{...props}
css={`
color: ${props.color};
`}
/>
Using the component above with a custom color
passed as a prop would look like this:
<Heading color='tomato'>
Hello
</Heading>
Note: unlike other libraries like styled-components, the css
prop takes a string, not a tagged template literal, so passing functions into the css
prop will do nothing.
Removing style props
To remove props used for styling from the underlying HTML element, use destructuring to pick out the props used for styling.
const Heading = ({ color, ...props }) =>
<Base.h2
{...props}
css={`
color: ${color};
`}
/>
Styling other components
To style other components, pass the component to the is
prop.
const Link = props =>
<Base
is={ReactRouter.Link}
{...props}
css={`
color: #07c;
`}
/>
Extending components
To make an Axs component extensible, pass the css
prop argument to the Base component's css
prop after the base styles.
const Heading = ({ css, ...props }) =>
<Base.h2
{...props}
css={`
font-size: 32px;
${css}
`}
/>
To make an extension from the base Heading component, pass it to the is
prop.
const BigHeading = props =>
<Base
{...props}
is={Heading}
css={`
font-size: 64px;
`}
/>
Server side rendering
Server side rendering works without the need for any additional setup.
This is because CSS rulesets are created within the Base component,
which then renders an inline <style>
element with the component's CSS.
To render in Node.js, be sure to use CommonJS module syntax or transpile ES modules to CommonJS syntax with a tool like Babel.
const React = require('react')
const { renderToString } = require('react-dom')
const Base = require('axs')
const html = renderToString(
React.createElement(Base, {
css: `
color: tomato;
`
}, 'Hello')
)
Pseudo-classes
Since Axs uses stylis to parse strings to valid CSS,
pseudo-classes can be added to components with a syntax like the following.
The &
refers to the component's generated className.
const Link = props =>
<Base.a
{...props}
css={`
color: #07c;
text-decoration: none;
&:hover {
text-decoration: underline;
}
`}
/>
Media queries
Media queries can also be handled inline with stylis syntax.
const Heading = props =>
<Base.h2
{...props}
css={`
font-size: 32px;
@media screen and (min-width: 40em) {
font-size: 48px;
}
`}
/>
Animation
CSS animations can also be handled with stylis syntax.
const Heading = props =>
<Base.h2
{...props}
css={`
font-size: 32px;
animation-name: pop;
animation-timing-function: ease-in;
animation-duration: .2s;
@keyframes pop {
0% { transform: scale(.75); }
50% { transform: scale(1.125); }
100% { transform: scale(1); }
}
`}
/>
Theming
Theming can be added to any Axs component using the theming library.
import React from 'react'
import Base from 'axs'
import { withTheme, ThemeProvider } from 'theming'
import theme from './theme'
const Heading = withTheme(({ color, theme, ...props }) =>
<Base.h2
{...props}
css={`
color: ${theme.blue};
`}
/>
)
const App = props => (
<ThemeProvider theme={theme}>
<Heading>
Hello
</Heading>
</ThemeProvider>
)
API
Base
The Base component is a primitive React component that takes CSS as a prop, generates a unique className,
and renders an inline style element along with the component.
The css
prop takes a string in a CSS-like syntax and uses stylis to create valid CSS rules.
By default the Base component renders an HTML <div>
element.
To change the underlying HTML element, pass a tag to the is
prop or use one of the keyed Base components.
<Base
is='h2'
css='color:tomato;'
/>
// using a Base component key
<Base.h2
css='color:tomato;'
/>
Prop | Type | Description |
---|---|---|
css |
String | stylis compatible CSS string to apply to the component |
is |
String or Component | sets the underlying HTML tag or React component to style |
innerRef |
Function | callback to get the component's React ref |
Motivation
React provides a powerful, functional API for rendering HTML with JavaScript. Creating styles for HTML can follow a similar pattern. By building on top of the React API, Axs is meant to make styling components effortless and require as little new syntax as possible. While other CSS-in-JS libraries add custom factory functions, methods, and additional utilities, Axs relies solely on React components and props for its API. The hope is that this makes Axs quicker to learn, easier to use, and more interoperable with the rest of the React ecosystem.
Axs also uses React to render inline style tags rather than adding custom DOM-related code, which makes using the library for server-side rendering work in the same way other React components do. This also means components can be rendered to other documents, such as in iframes, without the need for custom APIs or additional functionality.