BlinkDL / Blinkdl
Licence: apache-2.0
A minimalist deep learning library in Javascript using WebGL + asm.js. Run convolutional neural network in your browser.
Stars: ✭ 69
Programming Languages
javascript
184084 projects - #8 most used programming language
Projects that are alternatives of or similar to Blinkdl
Faceswap
Deepfakes Software For All
Stars: ✭ 39,911 (+57742.03%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Ssd Pytorch
SSD: Single Shot MultiBox Detector pytorch implementation focusing on simplicity
Stars: ✭ 107 (+55.07%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Deepfacelab
DeepFaceLab is the leading software for creating deepfakes.
Stars: ✭ 30,308 (+43824.64%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Bidaf Keras
Bidirectional Attention Flow for Machine Comprehension implemented in Keras 2
Stars: ✭ 60 (-13.04%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Deep Kernel Gp
Deep Kernel Learning. Gaussian Process Regression where the input is a neural network mapping of x that maximizes the marginal likelihood
Stars: ✭ 58 (-15.94%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Paddlex
PaddlePaddle End-to-End Development Toolkit(『飞桨』深度学习全流程开发工具)
Stars: ✭ 3,399 (+4826.09%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Quickdraw
Implementation of Quickdraw - an online game developed by Google
Stars: ✭ 805 (+1066.67%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Machine Learning Tutorials
machine learning and deep learning tutorials, articles and other resources
Stars: ✭ 11,692 (+16844.93%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Awesome Deep Learning Music
List of articles related to deep learning applied to music
Stars: ✭ 2,195 (+3081.16%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Deeplearning4j
All DeepLearning4j projects go here.
Stars: ✭ 68 (-1.45%)
Mutual labels: deep-neural-networks, neural-networks, deeplearning
Bmw Tensorflow Training Gui
This repository allows you to get started with a gui based training a State-of-the-art Deep Learning model with little to no configuration needed! NoCode training with TensorFlow has never been so easy.
Stars: ✭ 736 (+966.67%)
Mutual labels: deep-neural-networks, deeplearning
Kur
Descriptive Deep Learning
Stars: ✭ 811 (+1075.36%)
Mutual labels: deep-neural-networks, neural-networks
Concise Ipython Notebooks For Deep Learning
Ipython Notebooks for solving problems like classification, segmentation, generation using latest Deep learning algorithms on different publicly available text and image data-sets.
Stars: ✭ 23 (-66.67%)
Mutual labels: deep-neural-networks, deeplearning
Basic reinforcement learning
An introductory series to Reinforcement Learning (RL) with comprehensive step-by-step tutorials.
Stars: ✭ 826 (+1097.1%)
Mutual labels: neural-networks, deeplearning
Neupy
NeuPy is a Tensorflow based python library for prototyping and building neural networks
Stars: ✭ 670 (+871.01%)
Mutual labels: deep-neural-networks, deeplearning
Easy Deep Learning With Allennlp
🔮Deep Learning for text made easy with AllenNLP
Stars: ✭ 32 (-53.62%)
Mutual labels: deep-neural-networks, neural-networks
Artificialintelligenceengines
Computer code collated for use with Artificial Intelligence Engines book by JV Stone
Stars: ✭ 35 (-49.28%)
Mutual labels: neural-networks, deeplearning
Ffdl
Fabric for Deep Learning (FfDL, pronounced fiddle) is a Deep Learning Platform offering TensorFlow, Caffe, PyTorch etc. as a Service on Kubernetes
Stars: ✭ 640 (+827.54%)
Mutual labels: deep-neural-networks, deeplearning
Servenet
Service Classification based on Service Description
Stars: ✭ 21 (-69.57%)
Mutual labels: deep-neural-networks, deeplearning
Dbn Cuda
GPU accelerated Deep Belief Network
Stars: ✭ 38 (-44.93%)
Mutual labels: deep-neural-networks, neural-networks
BlinkDL
A minimalist deep learning library in Javascript using WebGL + asm.js. Runs in your browser.
Currently it is a proof-of-concept (inference only). Note: Convolution is buggy when memories overlap.
The WebGL backend is powered by weblas: https://github.com/waylonflinn/weblas.
Example
https://withablink.coding.me/goPolicyNet/ : a weiqi (baduk, go) policy network in AlphaGo style:
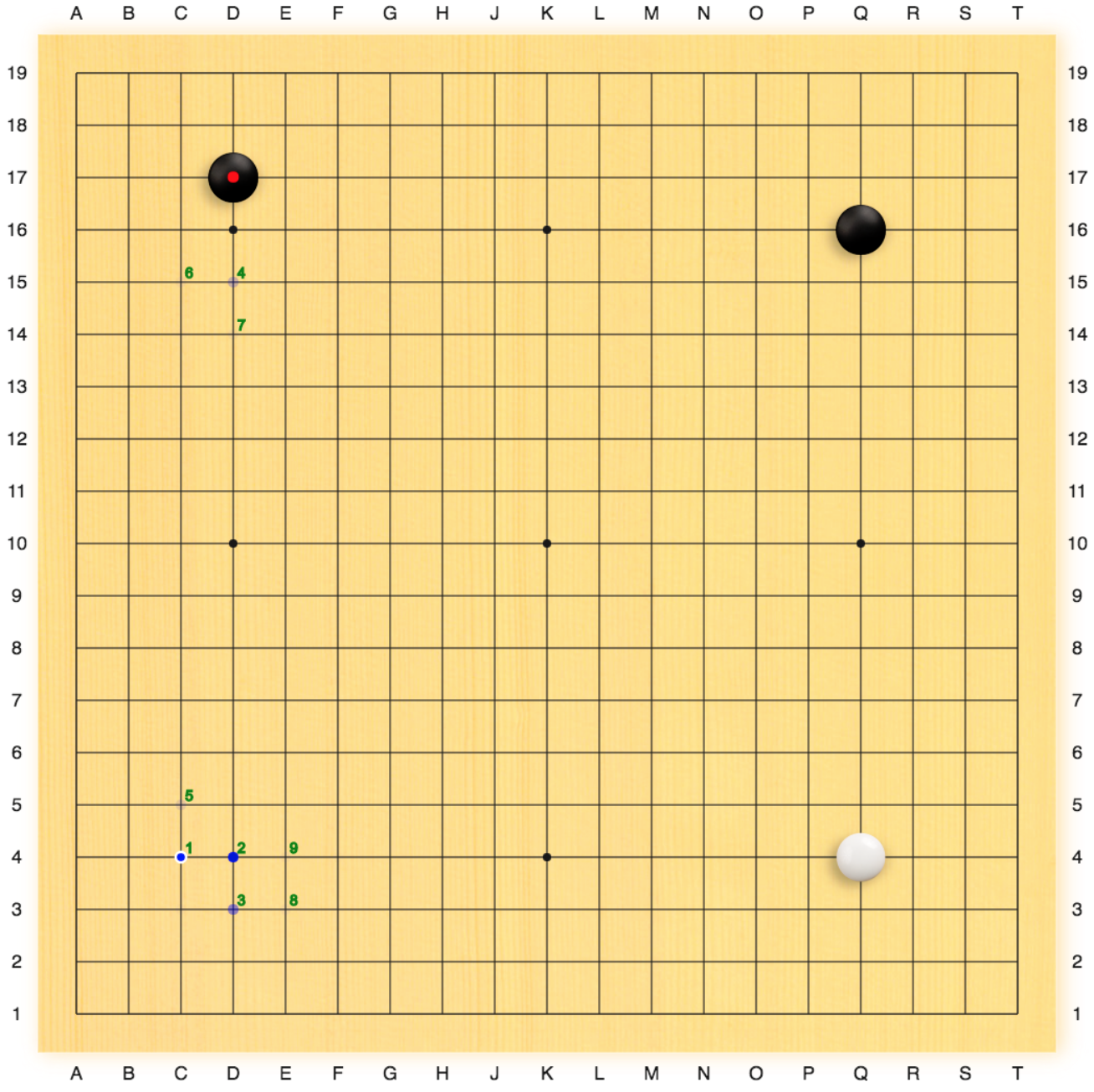
const N = 19;
const NN = N * N;
const nFeaturePlane = 8;
const nFilter = 128;
const x = new BlinkArray();
x.Init('weblas');
x.nChannel = nFeaturePlane;
x.data = new Float32Array(nFeaturePlane * NN);
for (var i = 0; i < NN; i++)
x.data[5 * NN + i] = 1; // set feature plane for empty board
// pre-act residual network with 6 residual blocks
const bak = new Float32Array(nFilter * NN);
x.Convolution(nFilter, 3);
x.CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak).CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak).CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak).CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak).CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak).CopyTo(bak);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.BatchNorm().ReLU().Convolution(nFilter, 3);
x.Add(bak);
x.BatchNorm().ReLU().Convolution(1, 1).Softmax();
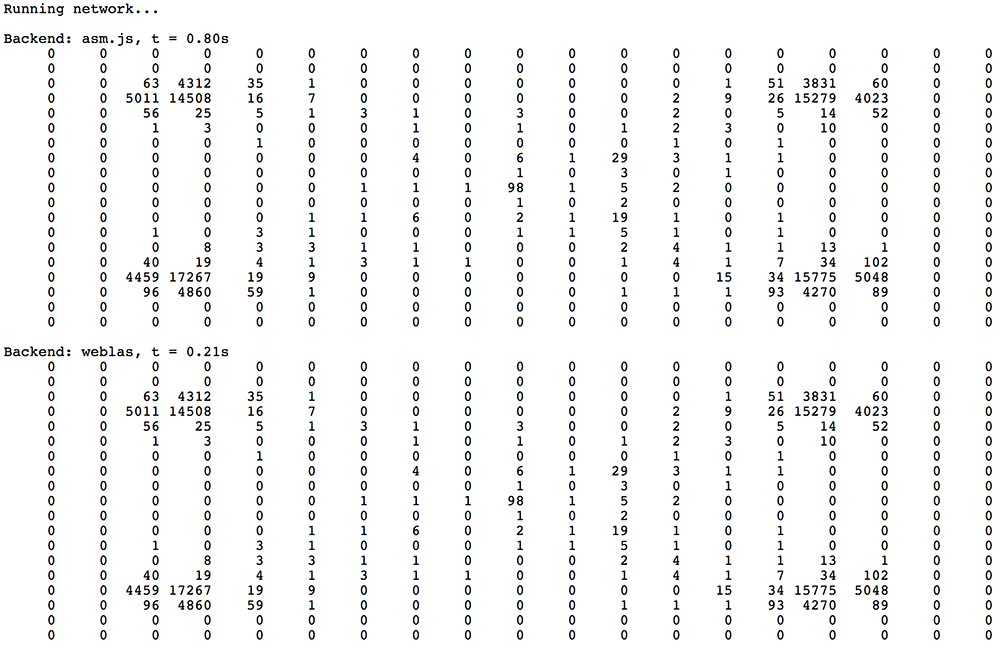
Usage
<script src='weblas.js' type='text/javascript'></script>
<script src='BlinkDL.js' type='text/javascript'></script>
Todo
- [x] Convolution (3x3_pad_1 and 1x1), BatchNorm, ReLU, Softmax
- [ ] Pooling layer
- [ ] FC layer
- [ ] Strided convolution
- [ ] Transposed convolution
- [ ] Webworker and async
- [ ] Faster inference with weblas pipeline, WebGPU, WebAssembly
- [ ] Memory manager
- [ ] Training
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].