ccwukong / Elfcommerce
Programming Languages
Projects that are alternatives of or similar to Elfcommerce
ElfCommerce
ElfCommerce is a headless ecommerce dashboard written in ReactJS + ExpressJS. Here is the developer guide for you to start with this project.
Demo account
Username: [email protected]
Password: 123
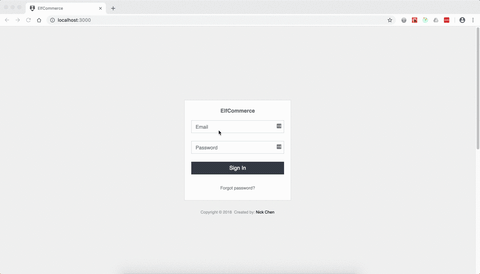
Installation
Step 1, clone this repo
Step 2, add the .env file in server directory with environment settings:
tokenSecret=REPLACE_THIS_WITH_ANY_LONG_RANDOM_STRING
dbHost=MYSQL_SERVER_CONNECTION_STRING
dbUser=MYSQL_USER
dbPassword=MYSQL_USER_PASSWORD
dbName=MYSQL_DATABASE_NAME
testDbName=MYSQL_DATABASE_NAME_FOR_INTEGRATION_TEST
sendgridApiKey=SENDGRID_API_KEY
sendgridDailyLimit=SENDGRID_DAILY_LIMIT_FOR_FREETIER
elasticemailApiKey=ELASTICEMAIL_API_KEY
elasticemailDailyLimit=ELASTICEMAIL_DAILY_LIMIT_FOR_FREETIER
passwordCallbackUrl=https://www.example.com
senderEmail=SYSTEM_EMAIL_SENDER_EMAIL
Step 3, install all dependancies for ExpressJS
cd server && yarn install
Step 4, install all dependancies for ReactJS
cd client && yarn install
Step 5, create your own config.js in client/src directory with following settings:
const config = {
apiDomain: 'API_DOMAIN',
accessTokenKey: 'THE_KEY_FOR_LOCAL_STORAGE_TO_STORE_ACCESS_TOKEN',
googleApiKey: 'GOOGLE_API_KEY',
mediaFileDomain: 'http://localhost:8080', //If you allow images to be uploaded to your local server
saveMediaFileLocal: false, //Set this to true if you allow images to be uploaded to your local server
sendgridApiKey: 'SENDGRID_API_KEY',
sendgridDailyLimit: 'SENDGRID_DAILY_LIMIT_FOR_FREETIER',
elasticemailApiKey: 'ELASTICEMAIL_API_KEY',
elasticemailDailyLimit: 'ELASTICEMAIL_DAILY_LIMIT_FOR_FREETIER',
passwordCallbackUrl: 'https://www.example.com',
senderEmail: 'SYSTEM_EMAIL_SENDER_EMAIL',
};
export default config;
Step 6, set up database
Before run the following command, make sure you already created a database and have it configured in your .env file.
cd server && yarn db:migrate
Step 7 (Optional), if you wanna deploy the RESTful API to AWS lambda function using ClaudiaJS, please make sure you follow the instructions.
ClaudiaJS doesn't create a Lambda function with environment variables from the .env file, thus you'll need to put all environment varibles in a .json file and run the following command when creating a Lambda function for the first time:
claudia create --handler lambda.handler --deploy-proxy-api --region AWS_REGION_NAME --set-env-from-json FILE_PATH
How to run this?
cd client && yarn start
Unit Test
For every main directory (components, containers etc.), there should be a __tests__ directory for all unit test cases.
cd clint && yarn test [test_directory]
cd server && yarn test [test_directory]
Project structure
Project restructured based on Fractal + ducks for greater scalability
βββ .circleci # CircleCI config file
βββ client # The web frontend written in ReactJS
β βββ public # Static public assets and uploads
β βββ src # ReactJS source code
β β βββ components # Shared components, like Button, Input etc.
β β β βββ __tests__ # Unit test for components
β β βββ pages # Top level components
β β β βββ __tests__ # Unit test for containers
β β β βββ ... # Sub components of top level components
β β βββ modules # Actions + Reducers using ducks file structure
β β β βββ __tests__ # Unit test for reducers
β β βββ utils # Utilities like language, date utils, string utils etc.
β β β βββ languages # All language translation .json files
β β β β βββ en.json # Language file
β β βββ App.css # Your customized styles should be added here
β β βββ App.js # ** Where React webapp routes configured.
β β βββ index.js # React webapp start point
β β βββ config.js # All global configurations(not included in this repo)
βββ scripts # Scaffolding & automation scripts
β βββ installation.js # Script to restore database, generate .env file etc.
βββ server # The web server part
β βββ db # Directory for database raw sql file, migration script etc.
β βββ exceptions # Directory for all API exception types
β βββ models # Directory for all API models
β β βββ tests # Directory for all API models test cases
β β βββ account.js # User model
β β βββ auth.js # Authentication model
β β βββ categorty.js # Category model
β β βββ index.js # Aggregates all model files
β β βββ manufacturer.js # Manufacturer model
β β βββ order.js # Order model
β β βββ product.js # Product model
β β βββ public.js # Public data model
β β βββ report.js # Report model
β β βββ store.js # Store model
β β βββ supplier.js # Supplier model
β β βββ vendor # For 3rd party modules
β βββ routes # Directory for all router files
β β βββ auth.js # Router for authentication endpoints
β β βββ category.js # Router for category endpoints
β β βββ common.js # Router for public data endpoints
β β βββ index.js # Aggregates all router files
β β βββ manufacturer.js # Router for manufacturer endpoints
β β βββ order.js # Router for order endpoints
β β βββ product.js # Router for product endpoints
β β βββ store.js # Router for store endpoints
β β βββ supplier.js # Router for supplier endpoints
β β βββ vendor # For 3rd party modules
β βββ uploads # Directory for image uploading, will be created automatically(not included in this repo)
β βββ .env # Global environment variables(not included in this repo)
β βββ app.js # Restful APIs written in ExpressJS
β βββ app.local.js # Wrapper file for claudia.js
β βββ lambda.js # Used by claudiajs for severless deployment, **Don't change contents here.
β βββ package.json # All project dependancies
β βββ middlewares.js # Middlewares for ExpressJS routes
βββ .eslintrc.json # **Don't change settings here.
βββ .prettierrc # **Don't change settings here.
βββ LICENSE # Project license file, **Don't change contents here.
βββ README.md # **Don't change contents here.
About the logo
Icons made by Freepik from www.flaticon.com is licensed by CC 3.0 BY
License
Elf Commerce is Apache-2.0 licensed.