francisrstokes / Hexnut
π© Hexnut is a middleware based, express/koa like framework for web sockets
Stars: β 204
Programming Languages
javascript
184084 projects - #8 most used programming language
Projects that are alternatives of or similar to Hexnut
Carrot
π₯ Build multi-device AR applications
Stars: β 32 (-84.31%)
Mutual labels: framework, websockets
Plezi
Plezi - the Ruby framework for realtime web-apps, websockets and RESTful HTTP
Stars: β 239 (+17.16%)
Mutual labels: framework, websockets
Circuits
circuits is a Lightweight Event driven and Asynchronous Application Framework for the Python Programming Language with a strong Component Architecture.
Stars: β 256 (+25.49%)
Mutual labels: framework, websockets
Uvicorn Gunicorn Starlette Docker
Docker image with Uvicorn managed by Gunicorn for high-performance Starlette web applications in Python 3.7 and 3.6 with performance auto-tuning. Optionally with Alpine Linux.
Stars: β 92 (-54.9%)
Mutual labels: framework, websockets
Facil.io
Your high performance web application C framework
Stars: β 1,393 (+582.84%)
Mutual labels: framework, websockets
Bolt Js
A framework to build Slack apps using JavaScript
Stars: β 1,971 (+866.18%)
Mutual labels: framework, websockets
Nest
A progressive Node.js framework for building efficient, scalable, and enterprise-grade server-side applications on top of TypeScript & JavaScript (ES6, ES7, ES8) π
Stars: β 42,981 (+20969.12%)
Mutual labels: framework, websockets
Http
An opinionated framework for scalable web π
Stars: β 136 (-33.33%)
Mutual labels: framework, websockets
Chimee
a video player framework aims to bring wonderful experience on browser
Stars: β 2,332 (+1043.14%)
Mutual labels: framework
Flamingo
Flamingo Framework and Core Library. Flamingo is a go based framework for pluggable web projects. It is used to build scalable and maintainable (web)applications.
Stars: β 198 (-2.94%)
Mutual labels: framework
Pydark
PyDark is a 2D and Online Multiplayer video game framework written on-top of Python and PyGame.
Stars: β 201 (-1.47%)
Mutual labels: framework
Antch
Antch, a fast, powerful and extensible web crawling & scraping framework for Go
Stars: β 198 (-2.94%)
Mutual labels: framework
Pgdeltastream
Streaming Postgres logical replication changes atleast-once over websockets
Stars: β 197 (-3.43%)
Mutual labels: websockets
Pest
Pest is an elegant PHP Testing Framework with a focus on simplicity
Stars: β 3,712 (+1719.61%)
Mutual labels: framework
Djangochannelsgraphqlws
Django Channels based WebSocket GraphQL server with Graphene-like subscriptions
Stars: β 203 (-0.49%)
Mutual labels: websockets
Framework
The liβ fullstack distribution, including overarching directory layout, starting application, and a copy of the framework.
Stars: β 199 (-2.45%)
Mutual labels: framework
Hexnut
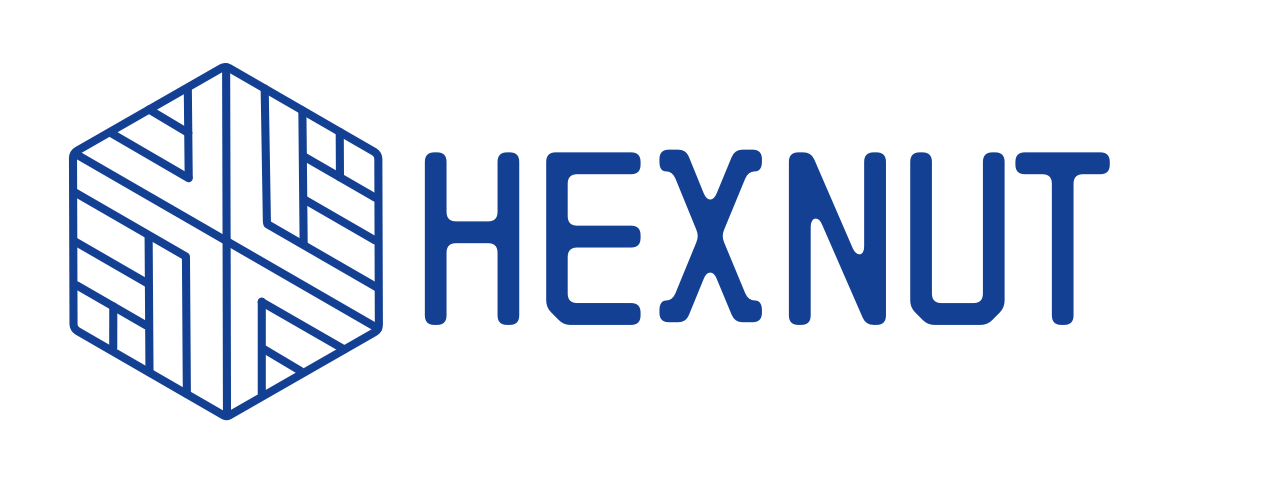
Hexnut is a middleware based, express/koa like framework for web sockets.
For an introduction, and API documentation, please check out the docs.
Middleware
- hexnut-handle: Basic middleware abstraction for handling connections and messages
- hexnut-bodyparser: Automatically parse JSON messages
- hexnut-sequence: Create sequenced conversations between client and server
-
hexnut-with-observable: Integration with
rxjs
- hexnut-restore-connection: Allow the client to restore a connection state if connectivity is lost
- hexnut-router: Respond differently when sockets connect and communicate on different URLs. Great for versioning!
Client side
You can use hexnut as a client in the frontend with hexnut-client
. It is also middleware based and can use many of the server middlewares directly, such as hexnut-bodyparser
and hexnut-sequence
.
Examples
Trivial Example
const Hexnut = require('hexnut');
const errorService = require(/* some error logging service */);
const app = new Hexnut({ port: 8080 });
app.onerror = async (err, ctx) => {
await errorService(err);
ctx.send(`Error! ${err.message}`);
};
app.use(ctx => {
if (ctx.isConnection) {
ctx.state = { count: 0 };
return ctx.send('Hello, and welcome to the socket!');
}
ctx.state.count++;
ctx.send(`Message No. ${ctx.state.count}: ${ctx.message}`);
});
app.start();
Parsing JSON automatically
const Hexnut = require('hexnut');
const bodyParser = require('hexnut-bodyparser');
const app = new Hexnut({ port: 8080 });
app.use(bodyParser.json());
app.use(ctx => {
if (ctx.isConnection) {
ctx.state = { count: 0 };
return ctx.send('Hello, and welcome to the socket!');
}
if (ctx.message.type) {
ctx.state.count++;
ctx.send(`Message No. ${ctx.state.count}: ${ctx.message.type}`);
} else {
ctx.send(`Invalid message format, expecting JSON with a "type" key`);
}
});
app.start();
Handling messages by type
const Hexnut = require('hexnut');
const handle = require('hexnut-handle');
const app = new Hexnut({ port: 8080 });
app.use(handle.connect(ctx => {
ctx.count = 0;
}));
app.use(handle.matchMessage(
msg => msg === 'incCount',
ctx => ctx.count++
));
app.use(handle.matchMessage(
msg => msg === 'decCount',
ctx => ctx.count--
));
app.use(handle.matchMessage(
msg => msg === 'getCount',
ctx => ctx.send(ctx.count)
));
app.start();
Sequencing Interactions
const Hexnut = require('hexnut');
const bodyParser = require('hexnut-bodyparser');
const sequence = require('hexnut-sequence');
const app = new Hexnut({ port: 8080 });
app.use(bodyParser.json());
// This sequence happens when the user connects
app.use(sequence.onConnect(function* (ctx) {
ctx.send(`Welcome, ${ctx.ip}`);
const name = yield sequence.getMessage();
ctx.clientName = name;
return;
}));
app.use(sequence.interruptible(function* (ctx) {
// In order to use this sequence, we assert that we must have a clientName on the ctx
yield sequence.assert(() => 'clientName' in ctx);
// We first expect a message with type == greeting
const greeting = yield sequence.matchMessage(msg => msg.type === 'greeting');
// Then a message that has type == timeOfDay
const timeOfDay = yield sequence.matchMessage(msg => msg.type === 'timeOfDay');
return ctx
.send(`And a ${greeting.value} to you too, ${ctx.clientName} on this fine ${timeOfDay.value}`);
}));
app.start();
Integrating with rxjs
const Hexnut = require('hexnut');
const { withObservable, filterMessages } = require('hexnut-with-observable');
const { tap, filter } = require('rxjs/operators');
const { pipe } = require('rxjs');
const app = new Hexnut({ port: 8181 });
app.use(withObservable(pipe(
// Do setup operations here...
tap(({ctx}) => {
if (ctx.isConnection) {
console.log(`[${ctx.ip}] Connection started`);
}
}),
// Filter to only messages
filterMessages(msg => msg !== 'skip'),
// Process messages
tap(({ctx, next}) => {
ctx.send(`You sent: ${ctx.message}`);
})
)));
app.start();
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].