nschloe / Meshio
Programming Languages
Projects that are alternatives of or similar to Meshio
I/O for mesh files.
There are various mesh formats available for representing unstructured meshes. meshio can read and write all of the following and smoothly converts between them:
Abaqus (
.inp
), ANSYS msh (.msh
), AVS-UCD (.avs
), CGNS (.cgns
), DOLFIN XML (.xml
), Exodus (.e
,.exo
), FLAC3D (.f3grid
), H5M (.h5m
), Kratos/MDPA (.mdpa
), Medit (.mesh
,.meshb
), MED/Salome (.med
), Nastran (bulk data,.bdf
,.fem
,.nas
), Neuroglancer precomputed format, Gmsh (format versions 2.2, 4.0, and 4.1,.msh
), OBJ (.obj
), OFF (.off
), PERMAS (.post
,.post.gz
,.dato
,.dato.gz
), PLY (.ply
), STL (.stl
), Tecplot .dat, TetGen .node/.ele, SVG (2D output only) (.svg
), SU2 (.su2
), UGRID (.ugrid
), VTK (.vtk
), VTU (.vtu
), WKT (TIN) (.wkt
), XDMF (.xdmf
,.xmf
).
Install with
pip install meshio[all]
([all]
pulls in all optional dependencies. By default, meshio only uses numpy.)
You can then use the command-line tools
meshio-convert input.msh output.vtk # convert between two formats
meshio-info input.xdmf # show some info about the mesh
meshio-compress input.vtu # compress the mesh file
meshio-decompress input.vtu # decompress the mesh file
meshio-binary input.msh # convert to binary format
meshio-ascii input.msh # convert to ASCII format
with any of the supported formats.
In Python, simply do
import meshio
mesh = meshio.read(
filename, # string, os.PathLike, or a buffer/open file
file_format="stl", # optional if filename is a path; inferred from extension
)
# mesh.points, mesh.cells, mesh.cells_dict, ...
# mesh.vtk.read() is also possible
to read a mesh. To write, do
import meshio
points = [
[0.0, 0.0, 0.0],
[0.0, 1.0, 0.0],
[0.0, 0.0, 1.0],
]
cells = [("triangle", [[0, 1, 2]])]
meshio.Mesh(
points,
cells
# Optionally provide extra data on points, cells, etc.
# point_data=point_data,
# cell_data=cell_data,
# field_data=field_data
).write(
"foo.vtk", # str, os.PathLike, or buffer/open file
# file_format="vtk", # optional if first argument is a path; inferred from extension
)
# Alternative with the same options
meshio.write_points_cells("foo.vtk", points, cells)
For both input and output, you can optionally specify the exact file_format
(in case you would like to enforce ASCII over binary VTK, for example).
Time series
The XDMF format supports time series with a shared mesh. You can write times series data using meshio with
with meshio.xdmf.TimeSeriesWriter(filename) as writer:
writer.write_points_cells(points, cells)
for t in [0.0, 0.1, 0.21]:
writer.write_data(t, point_data={"phi": data})
and read it with
with meshio.xdmf.TimeSeriesReader(filename) as reader:
points, cells = reader.read_points_cells()
for k in range(reader.num_steps):
t, point_data, cell_data = reader.read_data(k)
ParaView plugin
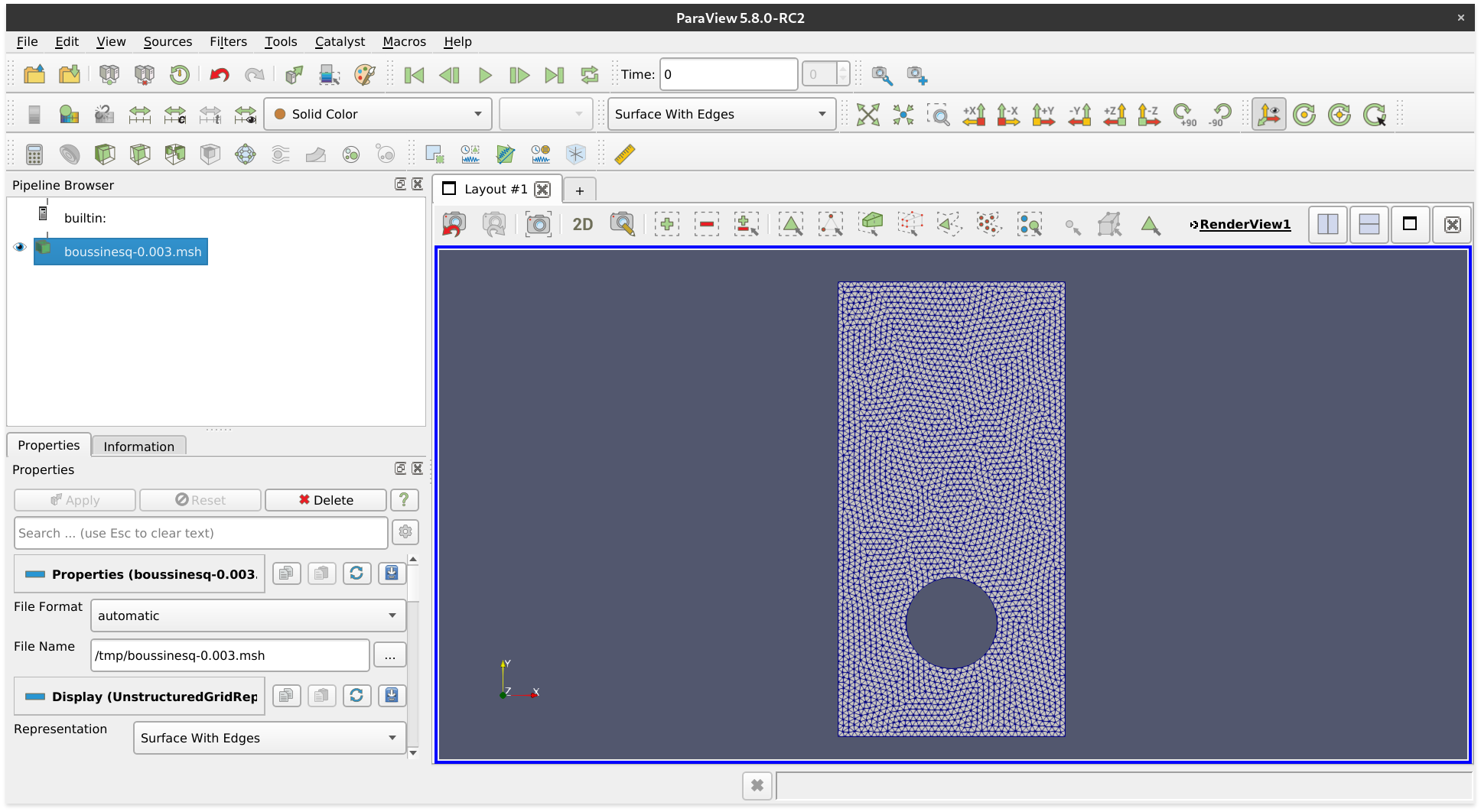
If you have downloaded a binary version of ParaView, you may proceed as follows.
- Make sure that ParaView uses a Python version that supports meshio. (That is at least Python 3.)
- Install meshio
- Open ParaView
- Find the file
paraview-meshio-plugin.py
of your meshio installation (on Linux:~/.local/share/paraview/plugins/
) and load it under Tools / Manage Plugins / Load New - Optional: Activate Auto Load
You can now open all meshio-supported files in ParaView.
Performance comparison
The comparisons here are for a triangular mesh with about 900k points and 1.8M triangles. The red lines mark the size of the mesh in memory.
File sizes
I/O speed
Maximum memory usage
Installation
meshio is available from the Python Package Index, so simply do
pip install meshio
to install.
Additional dependencies (netcdf4
, h5py
) are required for some of the output formats
and can be pulled in by
pip install meshio[all]
You can also install meshio from Anaconda:
conda install -c conda-forge meshio
Testing
To run the meshio unit tests, check out this repository and type
pytest
License
meshio is published under the MIT license.