lifenautjoe / Noel
Programming Languages
Projects that are alternatives of or similar to Noel
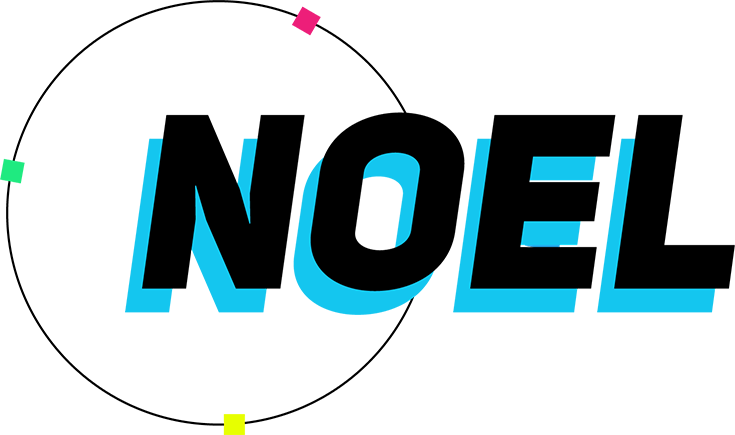
A universal, human-centric, replayable event emitter.
Table of Contents
- Motivation
- Features
- Installation
-
Usage
- Creating a noel instance
- Emitting and listening to events
- Replaying events
- Disabling replay
- Enabling replay
- Removing an event listener
- Removing all event listeners
- Clearing an event replay buffer
- Clearing all events replay buffers
- Changing the events replay buffer size
- Disabling the no event listeners warning
- Enabling the no event listeners warning
- Development
Motivation
The world just like software is full of events. Sometimes these events occur while we are busy doing other things. Wouldn't it be nice to have a way to replay all events? Noel is the way.
By being able to replay events we can design reactive systems without having to worry about timing.
For example, code like
// Check if there is a user in case we missed the userChanged event
const user = sessionService.getUser();
if(user) updateAvatarPhoto(user)
// Listen for further changes
sessionService.listenUserChanged(updateAvatarPhoto);
can become
sessionService.listenUserChanged(doSomethingWithUser).replay();
Meaning that if the userChanged
event was already fired, it will be replayed with the last arguments and if it gets fired again, we'll be listening.
Features
- Event replaying. Never miss a beat.
- API designed for humans. No useless concepts to try to make sense of.
Installation
npm install noel
Usage
Creating a noel instance
const Noel = require('Noel');
const noel = new Noel();
Emitting and listening to events
// Listen for an event
noel.on('friday', partyAllNightLong);
// Emit an event
noel.emit('friday', arg1, arg2 ....);
Replaying events
// Replay an event once
noel.on(eventName, eventListener).replay();
// Replay an event x amount of times
noel.on(eventName, eventListener).replay(x);
Disabling replay
// When creating the noel instance
const noel = new Noel({
replay: false
});
// At runtime
noel.disableReplay(anotherBufferSize);
Enabling replay
Please do note that replay is enabled by default, so this should only be necessary if it was disabled at runtime.
// At runtime
noel.enableReplay(anotherBufferSize);
Removing an event listener
noel.removeListener(eventName, eventListener);
or if you save a reference to the eventManager
const eventManager = noel.on('myEvent', eventListener);
// ...
eventManager.remove();
Removing all event listeners
noel.removeAllListeners(eventName, eventListener);
Clearing an event replay buffer
If you would like to clear all saved event emissions for replay
noel.clearReplayBufferForEvent(eventName);
Clearing all events replay buffers
If you would like to clear all saved events emissions for replay
noel.clearEventsReplayBuffers();
Changing the events replay buffer size
Or in human words, changing the amount of event emissions that are saved for replays.
Default is 1.
// When creating the noel instance
const noel = new Noel({
replayBufferSize: aNewBufferSize
});
// ...
// At runtime
noel.setReplayBufferSize(anotherBufferSize);
Disabling the no event listeners warning
When an event is emitted, no listeners have been set AND the replay was disabled so there is no way of doing anything with the emission, a warning will be logged into the console if available. To disable this behaviour:
// When creating the noel instance
const noel = new Noel({
noEventListenersWarning: false
});
// ...
// At runtime
noel.disableNoEventListenersWarning();
Enabling the no event listeners warning
Please do note that the no event listeners warning is enabled by default, so this should only be necessary if it was disabled at runtime.
noel.enableNoEventListenersWarning();
Development
Clone the repository
git clone [email protected]:lifenautjoe/noel.git
Use npm commands
-
npm t
: Run test suite -
npm start
: Runsnpm run build
in watch mode -
npm run test:watch
: Run test suite in interactive watch mode -
npm run test:prod
: Run linting and generate coverage -
npm run build
: Generate bundles and typings, create docs -
npm run lint
: Lints code -
npm run commit
: Commit using conventional commit style (husky will tell you to use it if you haven't 😉)
Author Joel Hernandez