prodo-dev / Prodo
Licence: mit
Prodo is a React framework to build apps faster.
Stars: ✭ 114
Programming Languages
typescript
32286 projects
Projects that are alternatives of or similar to Prodo
Flutter Boilerplate Project
A boilerplate project created in flutter using MobX and Provider.
Stars: ✭ 1,194 (+947.37%)
Mutual labels: boilerplate, state-management, mobx
Blue Chip
Normalizes GraphQL and JSON:API payloads into your state management system and provides ORM selectors to prepare data to be consumed by components
Stars: ✭ 332 (+191.23%)
Mutual labels: state-management, mobx
React Coat
Structured React + Redux with Typescript and support for isomorphic rendering beautifully(SSR)
Stars: ✭ 290 (+154.39%)
Mutual labels: state-management, mobx
Ignite Bowser
Bowser is now re-integrated into Ignite CLI! Head to https://github.com/infinitered/ignite to check it out.
Stars: ✭ 586 (+414.04%)
Mutual labels: boilerplate, mobx
micro-observables
A simple Observable library that can be used for easy state management in React applications.
Stars: ✭ 78 (-31.58%)
Mutual labels: state-management, mobx
Mobx Keystone
A MobX powered state management solution based on data trees with first class support for Typescript, support for snapshots, patches and much more
Stars: ✭ 284 (+149.12%)
Mutual labels: state-management, mobx
React Mobx React Router4 Boilerplate
React, React-Router 4, MobX and Webpack 2-boilerplate with async routes.
Stars: ✭ 566 (+396.49%)
Mutual labels: boilerplate, mobx
NObservable
MobX like observable state management library with Blazor support
Stars: ✭ 66 (-42.11%)
Mutual labels: state-management, mobx
React Mobx React Router Boilerplate
A simple boilerplate based on create-react-app and add mobx, react-router, linter, prettier and so on. 一个简单的 react 脚手架依赖于 create-react-app 新增了 mobx react-router,linter,prettier 等。
Stars: ✭ 12 (-89.47%)
Mutual labels: boilerplate, mobx
M Fe Boilerplates
Lucid & Futuristic Production Boilerplates For Frontend(Web) Apps, React/RN/Vue, with TypeScript(Optional), Webpack 4/Parcel, MobX/Redux 💫 多技术栈前端项目模板
Stars: ✭ 877 (+669.3%)
Mutual labels: boilerplate, mobx
react-coat-ssr-demo
Demo with Typescript + React + Redux for server-side-rendering (SSR)
Stars: ✭ 100 (-12.28%)
Mutual labels: state-management, mobx
Reactstatemuseum
A whirlwind tour of React state management systems by example
Stars: ✭ 1,294 (+1035.09%)
Mutual labels: state-management, mobx
hooksy
Simple app state management based on react hooks
Stars: ✭ 58 (-49.12%)
Mutual labels: state-management, mobx
Datx
DatX is an opinionated JS/TS data store. It features support for simple property definition, references to other models and first-class TypeScript support.
Stars: ✭ 111 (-2.63%)
Mutual labels: state-management, mobx
mutable
State containers with dirty checking and more
Stars: ✭ 32 (-71.93%)
Mutual labels: state-management, mobx
React Mobx Typescript Boilerplate
A bare minimum frontend boilerplate with React 16.7, Typescript 3.2 and Webpack 4
Stars: ✭ 378 (+231.58%)
Mutual labels: boilerplate, mobx
mst-effect
💫 Designed to be used with MobX-State-Tree to create asynchronous actions using RxJS.
Stars: ✭ 19 (-83.33%)
Mutual labels: state-management, mobx
ngx-mobx
Mobx decorators for Angular Applications
Stars: ✭ 14 (-87.72%)
Mutual labels: state-management, mobx
Mobx State Tree
Full-featured reactive state management without the boilerplate
Stars: ✭ 6,317 (+5441.23%)
Mutual labels: state-management, mobx
Compare React State Management
React createContext vs Apollo vs MobX vs Redux in a simple todo app.
Stars: ✭ 81 (-28.95%)
Mutual labels: state-management, mobx
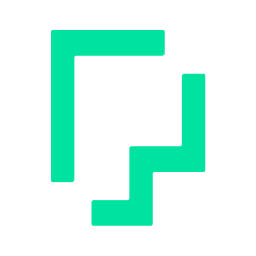
Prodo
Prodo is a React framework to write performant and scalable web apps with as little boilerplate as possible. View the Documentation. Read more about the motivation behind Prodo on our blog. Join us on Slack!
Key benefits
- 🎉 Drastically simplified state management
- ✨ Absolutely minimal boilerplate, especially compared to Redux
- ⚡️ Blazingly fast re-rendering, similar to MobX
- 👯♀️ Handles async actions out of the box
- 🔎 First class support for TypeScript
- ✅ Easily testable
- 🚀 Less to learn and shorter ramp up time
- 💪 Powerful plugins for routing, local storage, authentication, database, and more...
Show me the code
Define your model:
// src/model.ts
import { createModel } from "@prodo/core";
interface State {
count: number;
}
export const model = createModel<State>();
export const { state, watch, dispatch } = model.ctx;
Use your state:
// src/index.tsx
import { model, state, dispatch, watch } from "./model";
const changeCount = (amount: number) => {
state.count += amount;
};
const App = () => (
<div>
<button onClick={() => dispatch(changeCount)(-1)}>-</button>
<h1>Count: {watch(state.count)}</h1>
<button onClick={() => dispatch(changeCount)(1)}>+</button>
</div>
);
const { Provider } = model.createStore({
initState: {
count: 0,
},
});
ReactDOM.render(
<Provider>
<App />
</Provider>,
document.getElementById("root"),
);
As you can see in the above example, the state type was used once and everything else is automatically inferred.
Examples
There are some examples on CodeSandbox that you can view and edit.
There are also many example apps that use Prodo in examples/
. We recommend
looking at.
- Small to-do app example:
examples/todo-app
- Larger kanban app example:
examples/kanban
Running an example
Navigate to the example directory. For example:
cd examples/todo-app
Install dependencies
yarn
Run development server
yarn start
Navigate to localhost:8080.
Some examples have tests. You can run the tests with
yarn test
How to help?
- Help us gauge interest by starring this repository if you like what you see!
- Give feedback by opening an issue.
- Contribute code by opening a PR. See CONTRIBUTING for information on how to contribute code to the project.
Where to go next?
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].