lqs / Sqlingo
Licence: mit
💥 A lightweight DSL & ORM which helps you to write SQL in Go.
Stars: ✠142
Projects that are alternatives of or similar to Sqlingo
Go Sqlbuilder
A flexible and powerful SQL string builder library plus a zero-config ORM.
Stars: ✠539 (+279.58%)
Mutual labels: orm, database, mysql
Maghead
The fastest pure PHP database framework with a powerful static code generator, supports horizontal scale up, designed for PHP7
Stars: ✠483 (+240.14%)
Mutual labels: orm, database, mysql
Sqlboiler
Generate a Go ORM tailored to your database schema.
Stars: ✠4,497 (+3066.9%)
Mutual labels: orm, database, mysql
Hunt Entity
An object-relational mapping (ORM) framework for D language (Similar to JPA / Doctrine), support PostgreSQL and MySQL.
Stars: ✠51 (-64.08%)
Mutual labels: orm, database, mysql
Gnorm
A database-first code generator for any language
Stars: ✠415 (+192.25%)
Mutual labels: orm, database, mysql
Goloquent
This repo no longer under maintenance, please go to https://github.com/si3nloong/sqlike
Stars: ✠16 (-88.73%)
Mutual labels: orm, database, mysql
Bookshelf
A simple Node.js ORM for PostgreSQL, MySQL and SQLite3 built on top of Knex.js
Stars: ✠6,252 (+4302.82%)
Mutual labels: orm, database, mysql
Crecto
Database wrapper and ORM for Crystal, inspired by Ecto
Stars: ✠325 (+128.87%)
Mutual labels: orm, database, mysql
Rel
💎 Modern Database Access Layer for Golang - Testable, Extendable and Crafted Into a Clean and Elegant API
Stars: ✠317 (+123.24%)
Mutual labels: orm, database, mysql
Jooq
jOOQ is the best way to write SQL in Java
Stars: ✠4,695 (+3206.34%)
Mutual labels: orm, database, mysql
Architect
A set of tools which enhances ORMs written in Python with more features
Stars: ✠320 (+125.35%)
Mutual labels: orm, database, mysql
Denodb
MySQL, SQLite, MariaDB, PostgreSQL and MongoDB ORM for Deno
Stars: ✠498 (+250.7%)
Mutual labels: orm, database, mysql
Wetland
A Node.js ORM, mapping-based. Works with MySQL, PostgreSQL, SQLite and more.
Stars: ✠261 (+83.8%)
Mutual labels: orm, database, mysql
Sequelizer
A GUI Desktop App for export sequelize models from database automatically.
Stars: ✠273 (+92.25%)
Mutual labels: orm, database, mysql
Typeorm
ORM for TypeScript and JavaScript (ES7, ES6, ES5). Supports MySQL, PostgreSQL, MariaDB, SQLite, MS SQL Server, Oracle, SAP Hana, WebSQL databases. Works in NodeJS, Browser, Ionic, Cordova and Electron platforms.
Stars: ✠26,559 (+18603.52%)
Mutual labels: orm, database, mysql
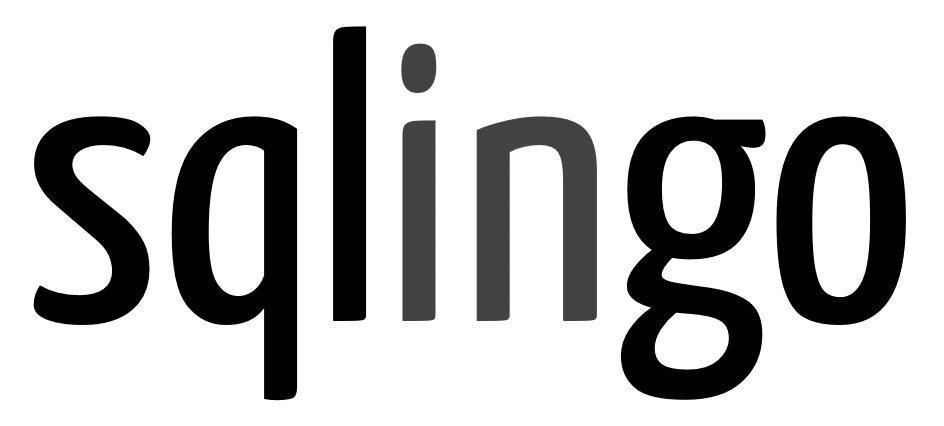
sqlingo is a SQL DSL (a.k.a. SQL Builder or ORM) library in Go. It generates code from the database and lets you write SQL queries in an elegant way.
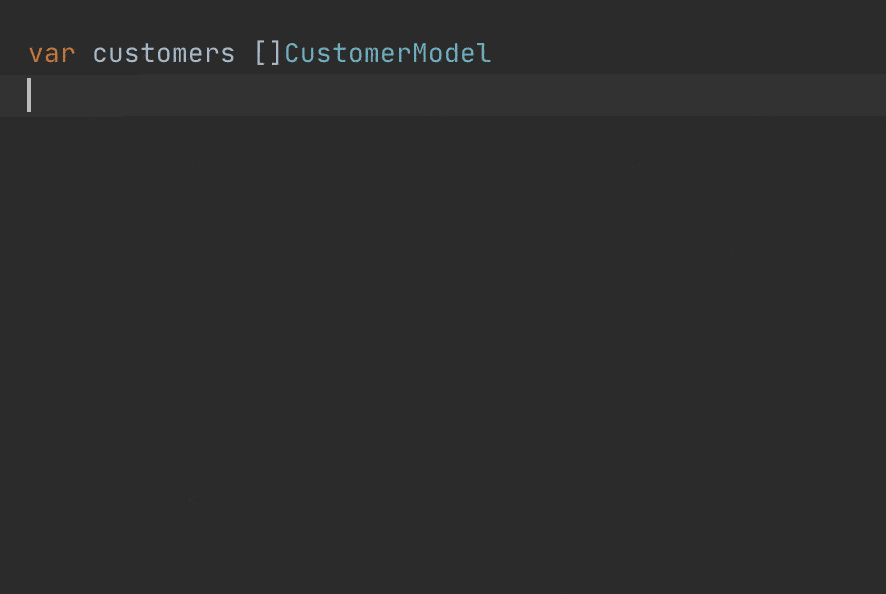
Features
- Auto-generating DSL objects and model structs from the database so you don't need to manually keep things in sync
- SQL DML (SELECT / INSERT / UPDATE / DELETE) with some advanced SQL query syntaxes
- Many common errors could be detected at compile time
- Your can use the features in your editor / IDE, such as autocompleting the fields and queries, or finding the usage of a field or a table
- Context support
- Transaction support
- Interceptor support
Database Support Status
Database | Status |
---|---|
MySQL | stable |
PostgreSQL | experimental |
SQLite | experimental |
Tutorial
Install and use sqlingo code generator
The first step is to generate code from the database. In order to generate code, sqlingo requires your tables are already created in the database.
$ go get -u github.com/lqs/sqlingo/sqlingo-gen-mysql
$ mkdir -p generated/sqlingo
$ sqlingo-gen-mysql root:[email protected]/database_name >generated/sqlingo/database_name.dsl.go
Write your application
Here's a demonstration of some simple & advanced usage of sqlingo.
package main
import (
"github.com/lqs/sqlingo"
. "./generated/sqlingo"
)
func main() {
db, err := sqlingo.Open("mysql", "root:[email protected]/database_name")
if err != nil {
panic(err)
}
// a simple query
var customers []*CustomerModel
db.SelectFrom(Customer).
Where(Customer.Id.In(1, 2)).
OrderBy(Customer.Name.Desc()).
FetchAll(&customers)
// query from multiple tables
var customerId int64
var orderId int64
err = db.Select(Customer.Id, Order.Id).
From(Customer, Order).
Where(Customer.Id.Equals(Order.CustomerId), Order.Id.Equals(1)).
FetchFirst(&customerId, &orderId)
// subquery and count
count, err := db.SelectFrom(Order)
Where(Order.CustomerId.In(db.Select(Customer.Id).
From(Customer).
Where(Customer.Name.Equals("Customer One")))).
Count()
// group-by with auto conversion to map
var customerIdToOrderCount map[int64]int64
err = db.Select(Order.CustomerId, f.Count(1)).
From(Order).
GroupBy(Order.CustomerId).
FetchAll(&customerIdToOrderCount)
if err != nil {
println(err)
}
// insert some rows
customer1 := &CustomerModel{name: "Customer One"}
customer2 := &CustomerModel{name: "Customer Two"}
_, err = db.InsertInto(Customer).
Models(customer1, customer2).
Execute()
// insert with on-duplicate-key-update
_, err = db.InsertInto(Customer).
Fields(Customer.Id, Customer.Name).
Values(42, "Universe").
OnDuplicateKeyUpdate().
Set(Customer.Name, Customer.Name.Concat(" 2")).
Execute()
}
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].