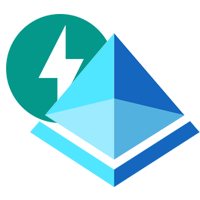
FastAPI-Azure-Auth
Azure AD Authentication for FastAPI apps made easy.
🚀 Description
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python, based on standard Python type hints.
At Intility we use FastAPI for both internal (single-tenant) and customer-facing (multi-tenant) APIs. This package enables our developers (and you
Also, we're hiring!
📚 Resources
The documentation contains a full tutorial on how to configure Azure AD and FastAPI for both single- and multi-tenant applications. It includes examples on how to lock down your APIs to certain scopes, tenants, roles etc. For first time users it's strongly advised to set up your application exactly how it's described there, and then alter it to your needs later.
MIT License | Documentation | GitHub
⚡ Setup
This is a tl;dr intended to give you an idea of what this package does and how to use it. For a more in-depth tutorial and settings reference you should read the documentation.
1. Install this library:
pip install fastapi-azure-auth
# or
poetry add fastapi-azure-auth
2. Configure your FastAPI app
Include swagger_ui_oauth2_redirect_url
and swagger_ui_init_oauth
in your FastAPI app initialization:
# file: main.py
app = FastAPI(
...
swagger_ui_oauth2_redirect_url='/oauth2-redirect',
swagger_ui_init_oauth={
'usePkceWithAuthorizationCodeGrant': True,
'clientId': settings.OPENAPI_CLIENT_ID,
},
)
3. Setup CORS
Ensure you have CORS enabled for your local environment, such as http://localhost:8000
.
4. Configure FastAPI-Azure-Auth
Configure either your SingleTenantAzureAuthorizationCodeBearer
or MultiTenantAzureAuthorizationCodeBearer
.
# file: demoproj/api/dependencies.py
from fastapi_azure_auth.auth import SingleTenantAzureAuthorizationCodeBearer
azure_scheme = SingleTenantAzureAuthorizationCodeBearer(
app_client_id=settings.APP_CLIENT_ID,
tenant_id=settings.TENANT_ID,
scopes={
f'api://{settings.APP_CLIENT_ID}/user_impersonation': 'user_impersonation',
}
)
or for multi-tenant applications:
# file: demoproj/api/dependencies.py
from fastapi_azure_auth.auth import MultiTenantAzureAuthorizationCodeBearer
azure_scheme = MultiTenantAzureAuthorizationCodeBearer(
app_client_id=settings.APP_CLIENT_ID,
scopes={
f'api://{settings.APP_CLIENT_ID}/user_impersonation': 'user_impersonation',
},
validate_iss=False
)
To validate the iss
, configure an
iss_callable
.
5. Configure dependencies
Add azure_scheme
as a dependency for your views/routers, using either Security()
or Depends()
.
# file: main.py
from demoproj.api.dependencies import azure_scheme
app.include_router(api_router, prefix=settings.API_V1_STR, dependencies=[Security(azure_scheme, scopes='user_impersonation')])
6. Load config on startup
Optional but recommended.
# file: main.py
@app.on_event('startup')
async def load_config() -> None:
"""
Load OpenID config on startup.
"""
await azure_scheme.openid_config.load_config()
📄 Example OpenAPI documentation
Your OpenAPI documentation will get an Authorize
button, which can be used to authenticate.
The user can select which scopes to authenticate with, based on your configuration.