gregnb / Filemanager Webpack Plugin
Programming Languages
Labels
Projects that are alternatives of or similar to Filemanager Webpack Plugin
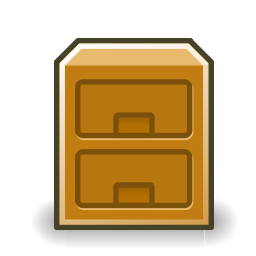
FileManager Webpack Plugin
This Webpack plugin allows you to copy, archive (.zip/.tar/.tar.gz), move, delete files and directories before and after builds
Install
npm install filemanager-webpack-plugin --save-dev
# or
yarn add filemanager-webpack-plugin --dev
Usage
// webpack.config.js:
const FileManagerPlugin = require('filemanager-webpack-plugin');
module.exports = {
plugins: [
new FileManagerPlugin({
events: {
onEnd: {
copy: [
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
{ source: '/path/**/*.js', destination: '/path' },
],
move: [
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
],
delete: ['/path/to/file.txt', '/path/to/directory/'],
mkdir: ['/path/to/directory/', '/another/directory/'],
archive: [
{ source: '/path/from', destination: '/path/to.zip' },
{ source: '/path/**/*.js', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip', format: 'tar' },
{
source: '/path/fromfile.txt',
destination: '/path/to.tar.gz',
format: 'tar',
options: {
gzip: true,
gzipOptions: {
level: 1,
},
globOptions: {
nomount: true,
},
},
},
],
},
},
}),
],
};
Options
new FileManagerPlugin({
events: {
onStart: {},
onEnd: {},
},
runTasksInSeries: false,
});
File Events
-
onStart
: Commands to execute before Webpack begins the bundling process
Note:
OnStart might execute twice for file changes in webpack context.
new webpack.WatchIgnorePlugin({
paths: [/copied-directory/],
});
-
onEnd
: Commands to execute after Webpack has finished the bundling process
File Actions
Copy
Copy individual files or entire directories from a source folder to a destination folder. Also supports glob pattern.
[
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/**/*.js', destination: '/path' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
{ source: '/path/**/*.{html,js}', destination: '/path/to' },
{ source: '/path/{file1,file2}.js', destination: '/path/to' },
];
Options
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file or a directory.
Caveats
- if source is a
glob
, destination must be a directory - if souce is a
file
and destination is a directory, the file will be copied into the directory
Delete
Delete individual files or entire directories. Also supports glob pattern
['/path/to/file.txt', '/path/to/directory/', '/another-path/to/directory/**.js'];
or
[
{
source: '/path/to/file.txt',
options: {
force: true,
},
},
];
Move
Move individual files or entire directories.
[
{ source: '/path/from', destination: '/path/to' },
{ source: '/path/fromfile.txt', destination: '/path/tofile.txt' },
];
Options
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file or a directory.
Mkdir
Create a directory path with given path
['/path/to/directory/', '/another/directory/'];
Archive
Archive individual files or entire directories. Defaults to .zip unless 'format' and 'options' provided. Uses node-archiver
[
{ source: '/path/from', destination: '/path/to.zip' },
{ source: '/path/**/*.js', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip' },
{ source: '/path/fromfile.txt', destination: '/path/to.zip', format: 'tar' },
{
source: '/path/fromfile.txt',
destination: '/path/to.tar.gz',
format: 'tar', // optional
options: {
// see https://www.archiverjs.com/archiver
gzip: true,
gzipOptions: {
level: 1,
},
globOptions: {
nomount: true,
},
},
},
];
- source[
string
] - a file or a directory or a glob - destination[
string
] - a file. - format[
string
] - Optional. Defaults to extension in destination filename. - options[
object
] - Refer https://www.archiverjs.com/archiver
Order of execution
If you need to preserve the order in which operations will run you can set the onStart and onEnd events to be Arrays. In this example below, in the onEnd event the copy action will run first, and then the delete after:
{
onEnd: [
{
copy: [{ source: './dist/bundle.js', destination: './newfile.js' }],
},
{
delete: ['./dist/bundle.js'],
},
];
}
Other Options
-
runTasksInSeries [
boolean
] - Run tasks in series. Defaults to false
For Example, the following will run one after the other
copy: [
{ source: 'dist/index.html', destination: 'dir1/' },
{ source: 'dir1/index.html', destination: 'dir2/' },
];
-
context [
string
] - The directory, an absolute path, for resolving files. Defaults to webpack context.