vitaviva / Fragivity
Licence: mit
Use Fragment like Activity
Stars: ✭ 143
Programming Languages
kotlin
9241 projects
Labels
Projects that are alternatives of or similar to Fragivity
Sdkmonitor
App to display and monitor the targetSDK from installed apps.
Stars: ✭ 122 (-14.69%)
Mutual labels: fragment
Androidall
Android 程序员需要掌握的技术栈:数据结构算法、程序架构、设计模式、性能优化、插件化、热更新、Kotlin、NDK、Jetpack,以及常用的开源框架源码分析如 Flutter、Router、RxJava、Glide、LeakCanary、Dagger2、Retrofit、OkHttp、ButterKnife 等
Stars: ✭ 849 (+493.71%)
Mutual labels: fragment
Thirtyinch
a MVP library for Android favoring a stateful Presenter
Stars: ✭ 1,052 (+635.66%)
Mutual labels: fragment
Neteasecloudmusic Mvvm
Jetpack MVVM最佳实践 - 重构仿网易云音乐安卓客户端
Stars: ✭ 103 (-27.97%)
Mutual labels: fragment
Compositeandroid
Composition over inheritance for Android components like Activity or Fragment
Stars: ✭ 519 (+262.94%)
Mutual labels: fragment
Navigator
Android Multi-module navigator, trying to find a way to navigate into a modularized android project
Stars: ✭ 131 (-8.39%)
Mutual labels: fragment
Rxbus
Android reactive event bus that simplifies communication between Presenters, Activities, Fragments, Threads, Services, etc.
Stars: ✭ 79 (-44.76%)
Mutual labels: fragment
Fragmentation
[DEPRECATED] A powerful library that manage Fragment for Android
Stars: ✭ 9,713 (+6692.31%)
Mutual labels: fragment
Android Progressfragment
Implementation of the fragment with the ability to display indeterminate progress indicator when you are waiting for the initial data.
Stars: ✭ 816 (+470.63%)
Mutual labels: fragment
Flowr
FlowR is a wrapper class around the Fragment Manager.
Stars: ✭ 123 (-13.99%)
Mutual labels: fragment
Androidnavigation
A library managing navigation, nested Fragment, StatusBar, Toolbar for Android
Stars: ✭ 636 (+344.76%)
Mutual labels: fragment
Fragnav
An Android library for managing multiple stacks of fragments
Stars: ✭ 1,379 (+864.34%)
Mutual labels: fragment
Rxlifecycle
Rx binding of stock Android Activities & Fragment Lifecycle, avoiding memory leak
Stars: ✭ 131 (-8.39%)
Mutual labels: fragment
Relay Example
[READONLY] 💝 Examples of common Relay patterns used in real-world applications. This repository is automatically exported from https://github.com/adeira/universe via Shipit
Stars: ✭ 126 (-11.89%)
Mutual labels: fragment
Androidxlazyload
😈😈Fragment lazy loading under the androix
Stars: ✭ 110 (-23.08%)
Mutual labels: fragment
Fragivity : Use Fragment like Activity
English | 中文文档
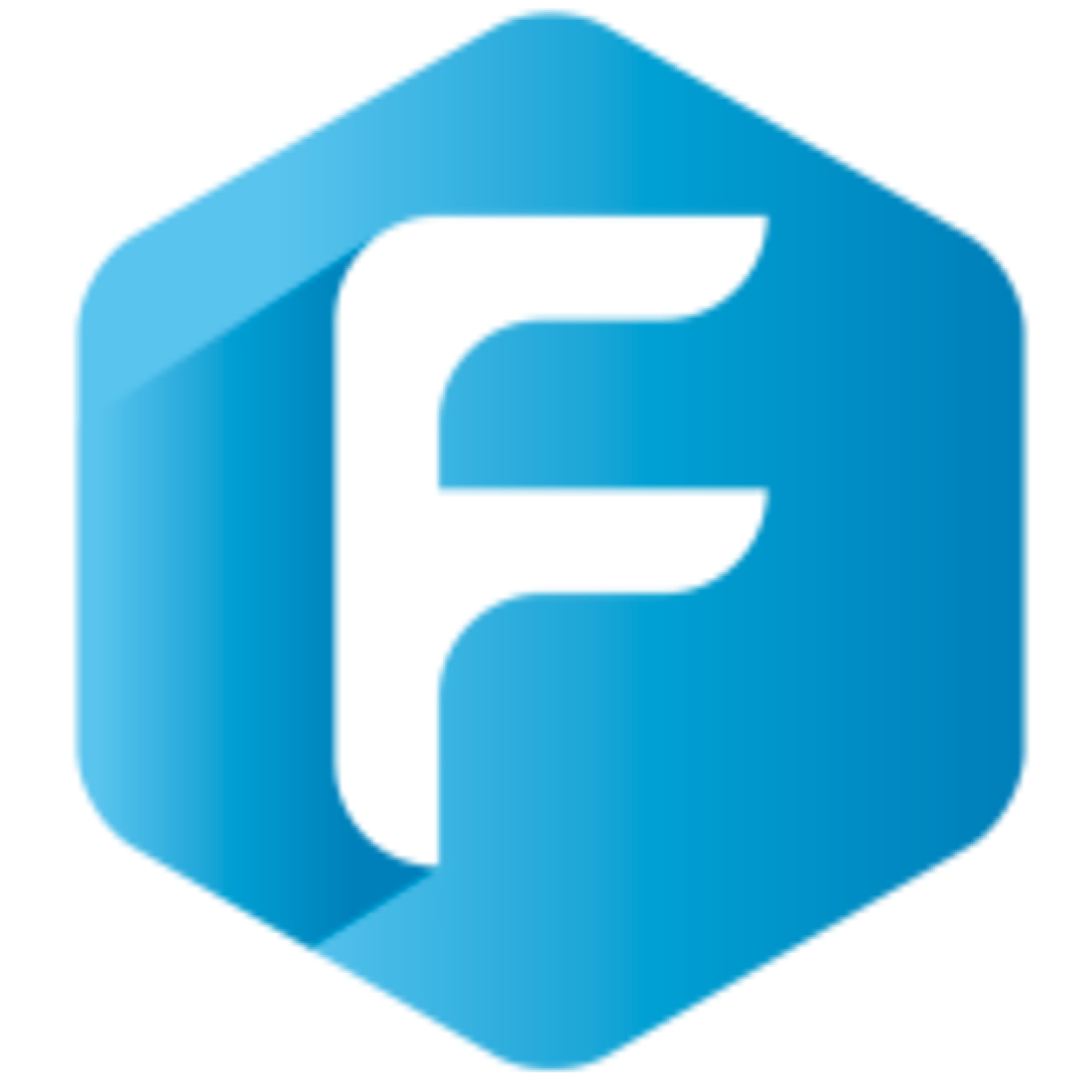
Fragivity is a library used to build APP with "Single Activity + Multi-Fragments" Architecture
- Reasonable Lifecycle: Lifecycle is consistent with Activity when screen changed
- Multiple LaunchModes: Supports multiple modes, such as Standard, SingleTop and SingleTask
- Transition animation: Supports Transition or SharedElement animation when switching screens
- Efficient communication: Simple and direct communication based on callback
- Friendly Backpress: Supports onBackPressed interception and SwipeBack
- Deep Links: Routes to the specified screen by URI
- Dialog: Supports DialogFragment
Installation
implementation 'com.github.fragivity:core:$latest_version'
Quick start
1. declare NavHostFragment in layout
Like Navigation
, Fragivity needs a NavHostFragment
as the host of ChildFragments
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:fitsSystemWindows="true">
<androidx.fragment.app.FragmentContainerView
android:id="@+id/nav_host"
android:name="androidx.navigation.fragment.NavHostFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:defaultNavHost="true" />
</FrameLayout>
2. load HomeFragment in Activity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val navHostFragment = supportFragmentManager
.findFragmentById(R.id.nav_host) as NavHostFragment
navHostFragment.loadRoot(HomeFragment::class)
//or loadRoot with factory
//navHostFragment.loadRoot{ HomeFragment() }
}
}
3. navigate to destination Fragment
//in HomeFragment
navigator.push(DestinationFragment::class) {
arguments = bundleOf(KEY_ARGUMENT1 to "arg1", KEY_ARGUMENT2 to "arg2")
//or applyArguments(KEY_ARGUMENT1 to "arg1", KEY_ARGUMENT2 to "arg2")
}
Launch Mode
Support multiple launch modes
navigator.push(DestinationFragment::class) {
launchMode = LaunchMode.STANDARD //default
//or LaunchMode.SINGLE_TOP, LaunchMode.SINGLE_TASK
}
Transition Animation
navigator.push(DestinationFragment::class) {
//animator
enterAnim = R.anim.slide_in
exitAnim = R.anim.slide_out
popEnterAnim = R.anim.slide_in_pop
popExitAnim = R.anim.slide_out_pop
//sharedElements
sharedElements = sharedElementsOf(imageView to "id")
}
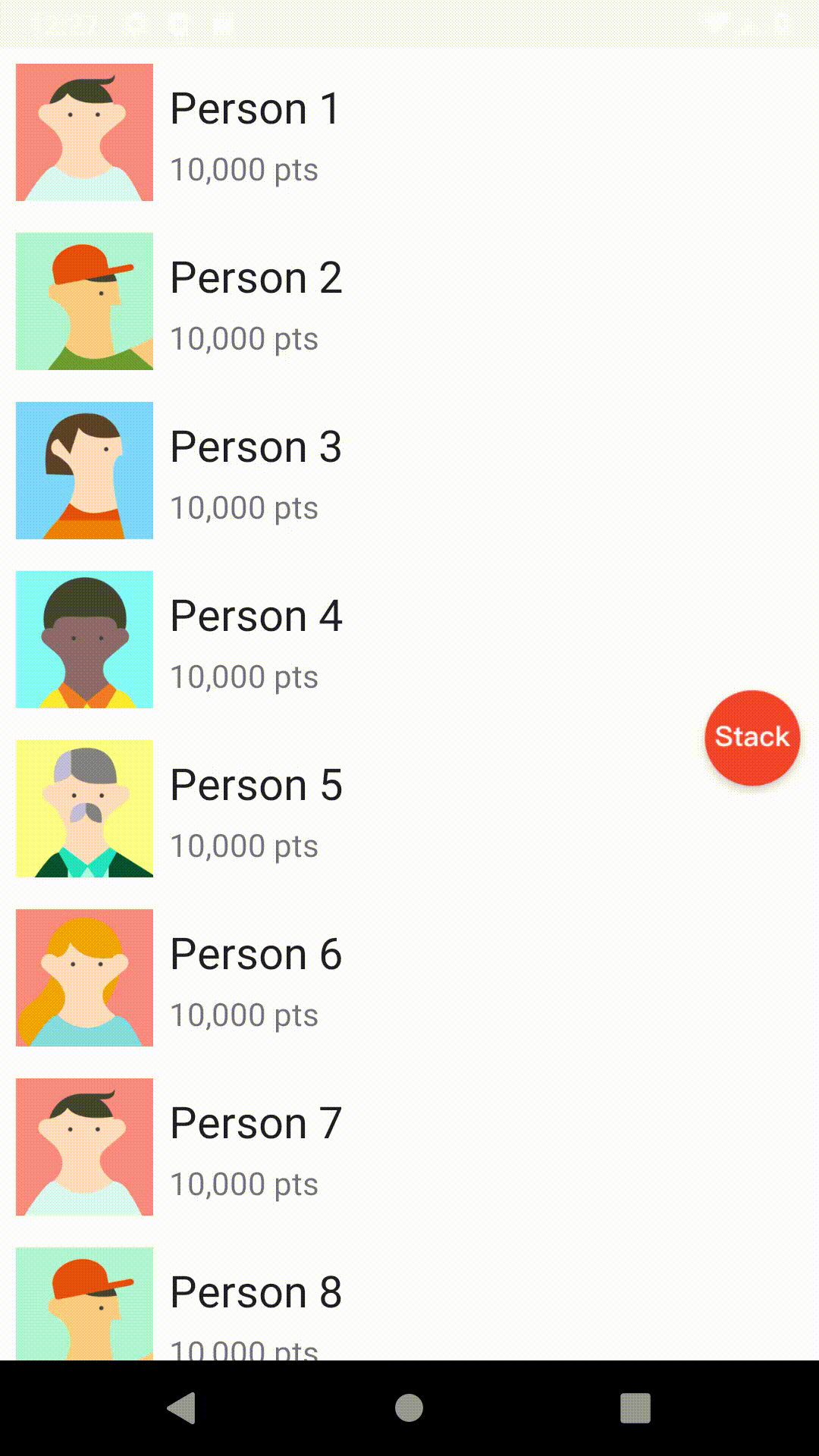
Communication
You can simply setup communication between two fragments
1. start destination Fragment with a callback
class HomeFragment : Fragment(){
private val cb: (Int) -> Unit = { checked ->
//...
}
//...
fun startDestination() {
navigator.push {
DestinationFragment(cb)
}
}
//...
}
2. callback to source Fragment
class DestinationFragment(val cb: (Int) -> Unit) : Fragment() {
//...
cb.invoke(xxx)
//...
}
Show Dialog
1. declare a DialogFragment
class DialogFragment : DialogFragment() {
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
val root = inflater.inflate(R.layout.fragment_dialog, container, false)
return root
}
}
2. show it
navigator.showDialog(DialogFragment::class)
Deep links
1. add kapt dependencies
kapt 'com.github.fragivity:processor:$latest_version'
@Deeplink
annotation
2. declare URI with @DeepLink(uri = "myapp://fragitiy.github.com/")
class DeepLinkFragment : Fragment() {
//...
}
3. handle intent in MainActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//...
navHostFragment.handleDeepLink(intent)
}
}
4. start Activity with URI
val intent = Intent(Intent.ACTION_VIEW, Uri.parse("myapp://fragitiy.github.com/"))
startActivity(intent)
Router
1.composable Fragment in Activity
with(navHostFragment) {
composable("feed") { FeedFragment.newInstance() }
composable("search?keyword={keyword}", stringArgument("keyword")) {
SearchFragment.newInstance()
}
}
2.navigate to destination Fragment
navigator.push("search?keyword=$value")
// or
navigator.push("search") {
arguments = bundleOf("keyword" to value.toString())
}
navigator.popTo("search")
Using in Java
Fragivity provides a set of APIs for Java developers
FAQ
License
Fragivity is licensed under the MIT License.
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].