rialto-php / Puphpeteer
Projects that are alternatives of or similar to Puphpeteer
PuPHPeteer
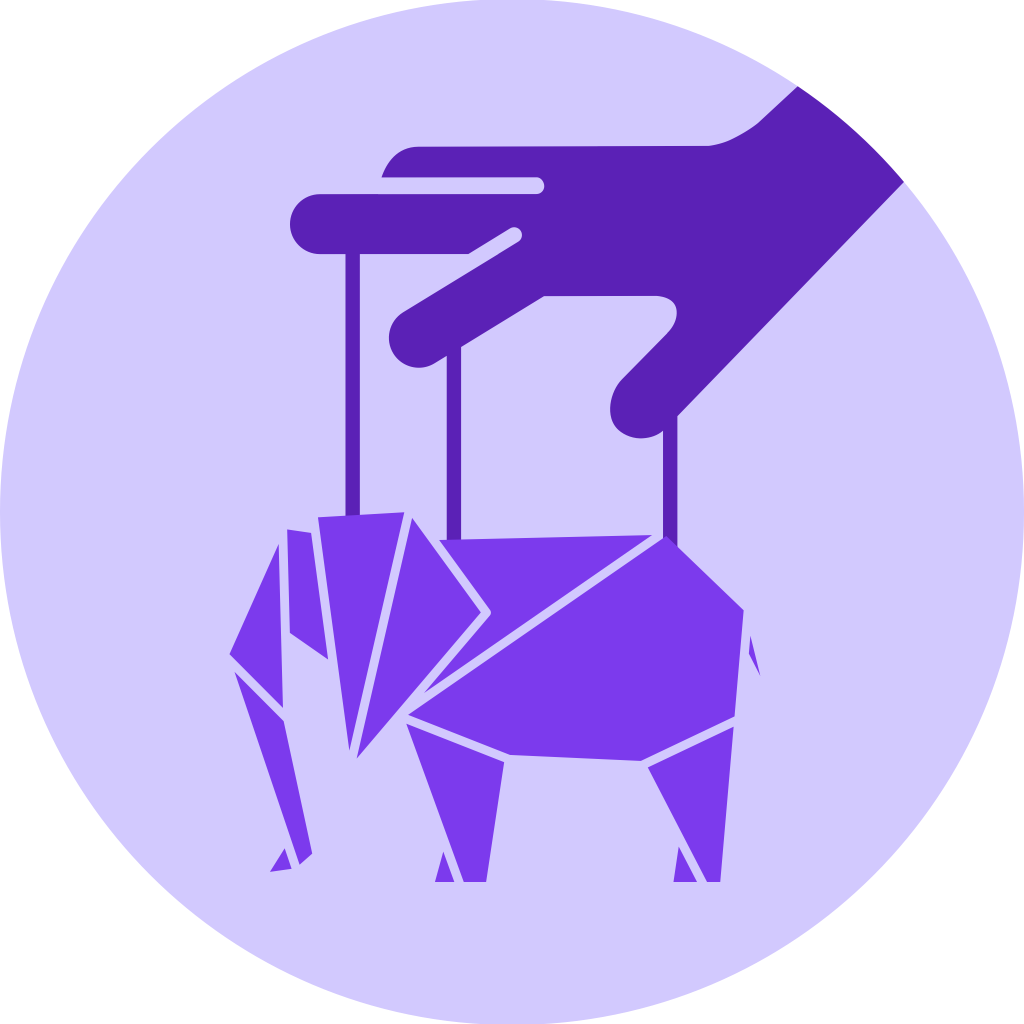
A Puppeteer bridge for PHP, supporting the entire API. Based on Rialto, a package to manage Node resources from PHP.
Here are some examples borrowed from Puppeteer's documentation and adapted to PHP's syntax:
Example - navigating to https://example.com and saving a screenshot as example.png:
use Nesk\Puphpeteer\Puppeteer;
$puppeteer = new Puppeteer;
$browser = $puppeteer->launch();
$page = $browser->newPage();
$page->goto('https://example.com');
$page->screenshot(['path' => 'example.png']);
$browser->close();
Example - evaluate a script in the context of the page:
use Nesk\Puphpeteer\Puppeteer;
use Nesk\Rialto\Data\JsFunction;
$puppeteer = new Puppeteer;
$browser = $puppeteer->launch();
$page = $browser->newPage();
$page->goto('https://example.com');
// Get the "viewport" of the page, as reported by the page.
$dimensions = $page->evaluate(JsFunction::createWithBody("
return {
width: document.documentElement.clientWidth,
height: document.documentElement.clientHeight,
deviceScaleFactor: window.devicePixelRatio
};
"));
printf('Dimensions: %s', print_r($dimensions, true));
$browser->close();
Requirements and installation
This package requires PHP >= 7.3 and Node >= 8.
Install it with these two command lines:
composer require nesk/puphpeteer
npm install @nesk/puphpeteer
Notable differences between PuPHPeteer and Puppeteer
Puppeteer's class must be instantiated
Instead of requiring Puppeteer:
const puppeteer = require('puppeteer');
You have to instantiate the Puppeteer
class:
$puppeteer = new Puppeteer;
This will create a new Node process controlled by PHP.
You can also pass some options to the constructor, see Rialto's documentation. PuPHPeteer also extends these options:
[
// Logs the output of Browser's console methods (console.log, console.debug, etc...) to the PHP logger
'log_browser_console' => false,
]
⏱ Want to use some timeouts higher than 30 seconds in Puppeteer's API?
If you use some timeouts higher than 30 seconds, you will have to set a higher value for the read_timeout
option (default: 35
):
$puppeteer = new Puppeteer([
'read_timeout' => 65, // In seconds
]);
$puppeteer->launch()->newPage()->goto($url, [
'timeout' => 60000, // In milliseconds
]);
await
keyword
No need to use the With PuPHPeteer, every method call or property getting/setting is synchronous.
Some methods have been aliased
The following methods have been aliased because PHP doesn't support the $
character in method names:
-
$
=>querySelector
-
$$
=>querySelectorAll
-
$x
=>querySelectorXPath
-
$eval
=>querySelectorEval
-
$$eval
=>querySelectorAllEval
Use these aliases just like you would have used the original methods:
$divs = $page->querySelectorAll('div');
JsFunction
Evaluated functions must be created with Functions evaluated in the context of the page must be written with the JsFunction
class, the body of these functions must be written in JavaScript instead of PHP.
use Nesk\Rialto\Data\JsFunction;
$pageFunction = JsFunction::createWithParameters(['element'])
->body("return element.textContent");
->tryCatch
Exceptions must be caught with If an error occurs in Node, a Node\FatalException
will be thrown and the process closed, you will have to create a new instance of Puppeteer
.
To avoid that, you can ask Node to catch these errors by prepending your instruction with ->tryCatch
:
use Nesk\Rialto\Exceptions\Node;
try {
$page->tryCatch->goto('invalid_url');
} catch (Node\Exception $exception) {
// Handle the exception...
}
Instead, a Node\Exception
will be thrown, the Node process will stay alive and usable.
License
The MIT License (MIT). Please see License File for more information.
Logo attribution
PuPHPeteer's logo is composed of:
- Puppet by Luis Prado from the Noun Project.
- Elephant by Lluisa Iborra from the Noun Project.
Thanks to Laravel News for picking the icons and colors of the logo.