unimonkiez / React Cordova Boilerplate
Programming Languages
Projects that are alternatives of or similar to React Cordova Boilerplate
React Cordova boilerplate
Demo - click the image to try it
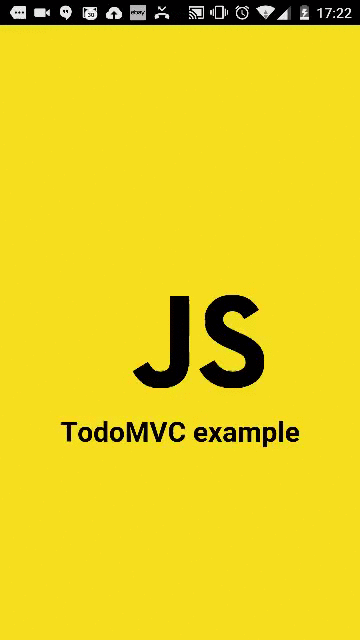
Why cordova and React
Cordova is really simple to build cross platform mobile applications for any of your needs, this boilerplate provides a great starting point for your next react project, and can be used to maintain a website and mobile application from same source code (and maybe transitioning later to react-native).
I highly advise to check out my other boilerplate, which is similar in many ways but produces a native app.
Features
- eslint
- Smart build using Webpack 2
- ES6
- React (jsx)
- Server rendering for initial page
- Style: Radium + SASS
- React router
- Testing
- Mocha
- jsdom (blazing fast testing on nodeJs)
- Sinon
- Chai
- Coverage using nyc
Installing
- Install dependencies:
npm i
oryarn install
- Install global tools:
npm install -g cordova
- Add your cordova platform by running
cordova platform add %PLATFORM%
(android and more)
Usage
-
npm run lint[:report]
- runs linting against src folder and fix some of the issues,report
option to generate html report to./report.html
. -
npm run start[:prod]
- starts a server, with react model replacement and devtools onlocalhost:8080
,prod
option to minify the build (same build eventually integrated with the cordova app). -
npm run build[:prod][:watch]
- builds the project (single html file and single js file) as it does for development. -
npm run test[:watch][:coverage]
- runs Mocha testing, outputs result to console,watch
options to watch files and test again after file modification,coverage
option to generate coverage reports to./coverage
folder (index.html
is a usuful one!).
Build and run as application
As you do with any cordova application, cordova build android
, cordova run android
and more.
cordova runs npm run build:prod
before any cordova command (using hooks).
Style practice
To style your html, simply inline style your DOM (here is why).
You can use Radium (which is included) to easily add 'css like' event listeners to your components (like hover).
Sass/CSS is included to complete some of the missing features or already written style you want to use. To use Sass/CSS simply import that file!
custom-font.scss
@font-face {
font-family: 'custom-font';
src:url('./custom-font.eot') format('embedded-opentype'),
url('./custom-font.ttf') format('truetype'),
url('./custom-font.woff') format('woff'),
url('./custom-font.svg') format('svg');
font-weight: normal;
font-style: normal;
}
.customFont {
font-family: 'custom-font';
&.customFontIcon {
content: "\e600";
&:hover {
color: blue;
}
}
}
ExampleComponent.jsx
import React, { Component } from 'react';
import customFont from './custom-font.scss'
export default class ExampleComponent extends Component {
render() {
return (
<div style={{backgroundColor: 'red'}}>
Hello world!
<span className={customFont.customFont + ' ' + customFont.customFontIcon}></span>
</div>
);
}
}
Advantages:
- Complete styling ability to go with inline style.
- Easily use third party styles.
- No globals - import style files and use the class (minifies signature - example
.a
instead of.customFont
)
Sass style will be minified, bundled and included to the server rendered file.
Troubleshooting
-
Node Sass could not find a binding for your current environment: OS X 64-bit with Node.js X.x
If you get an error like this means you previously installed Node SASS in an older version of Node which no longer applies to you. To fix runnpm rebuild node-sass