dogo / Sketchkit
Licence: mit
A lightweight auto-layout DSL library for iOS & tvOS.
Stars: ✭ 40
Projects that are alternatives of or similar to Sketchkit
Aksidemenu
Beautiful iOS side menu library with parallax effect. Written in Swift
Stars: ✭ 216 (+440%)
Mutual labels: cocoapods, carthage, swift-package-manager, spm
Sablurimageview
You can use blur effect and it's animation easily to call only two methods.
Stars: ✭ 538 (+1245%)
Mutual labels: cocoapods, carthage, swift-package-manager
Sica
🦌 Simple Interface Core Animation. Run type-safe animation sequencially or parallelly
Stars: ✭ 980 (+2350%)
Mutual labels: cocoapods, carthage, swift-package-manager
Zephyr
Effortlessly synchronize UserDefaults over iCloud.
Stars: ✭ 722 (+1705%)
Mutual labels: cocoapods, carthage, swift-package-manager
Openssl
OpenSSL package for SPM, CocoaPod, and Carthage, for iOS and macOS
Stars: ✭ 515 (+1187.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Swiftframeworktemplate
A template for new Swift iOS / macOS / tvOS / watchOS Framework project ready with travis-ci, cocoapods, Carthage, SwiftPM and a Readme file
Stars: ✭ 527 (+1217.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Validatedpropertykit
Easily validate your Properties with Property Wrappers 👮
Stars: ✭ 701 (+1652.5%)
Mutual labels: cocoapods, carthage, spm
Gzipswift
Swift framework that enables gzip/gunzip Data using zlib
Stars: ✭ 356 (+790%)
Mutual labels: cocoapods, carthage, spm
Statusalert
Display Apple system-like self-hiding status alerts. It is well suited for notifying user without interrupting user flow in iOS-like way.
Stars: ✭ 809 (+1922.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Preferences
⚙ Add a preferences window to your macOS app in minutes
Stars: ✭ 898 (+2145%)
Mutual labels: cocoapods, carthage, swift-package-manager
Swiftlyext
SwiftlyExt is a collection of useful extensions for Swift 3 standard classes and types 🚀
Stars: ✭ 31 (-22.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Keyboardshortcuts
Add user-customizable global keyboard shortcuts to your macOS app in minutes
Stars: ✭ 500 (+1150%)
Mutual labels: cocoapods, carthage, swift-package-manager
Tweetextfield
Lightweight set of text fields with nice animation and functionality. 🚀 Inspired by https://uimovement.com/ui/2524/input-field-help/
Stars: ✭ 421 (+952.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Bow
🏹 Bow is a cross-platform library for Typed Functional Programming in Swift
Stars: ✭ 538 (+1245%)
Mutual labels: cocoapods, carthage, spm
Simpleimageviewer
A snappy image viewer with zoom and interactive dismissal transition.
Stars: ✭ 408 (+920%)
Mutual labels: cocoapods, carthage, spm
Guitar
A Cross-Platform String and Regular Expression Library written in Swift.
Stars: ✭ 641 (+1502.5%)
Mutual labels: cocoapods, carthage, swift-package-manager
Swiftysound
SwiftySound is a simple library that lets you play sounds with a single line of code.
Stars: ✭ 995 (+2387.5%)
Mutual labels: cocoapods, carthage, spm
Functionkit
A framework for functional types and operations designed to fit naturally into Swift.
Stars: ✭ 302 (+655%)
Mutual labels: cocoapods, carthage, swift-package-manager
Misterfusion
MisterFusion is Swift DSL for AutoLayout. It is the extremely clear, but concise syntax, in addition, can be used in both Swift and Objective-C. Support Safe Area and Size Class.
Stars: ✭ 314 (+685%)
Mutual labels: cocoapods, carthage, autolayout
Defaults
Swifty and modern UserDefaults
Stars: ✭ 734 (+1735%)
Mutual labels: cocoapods, carthage, swift-package-manager
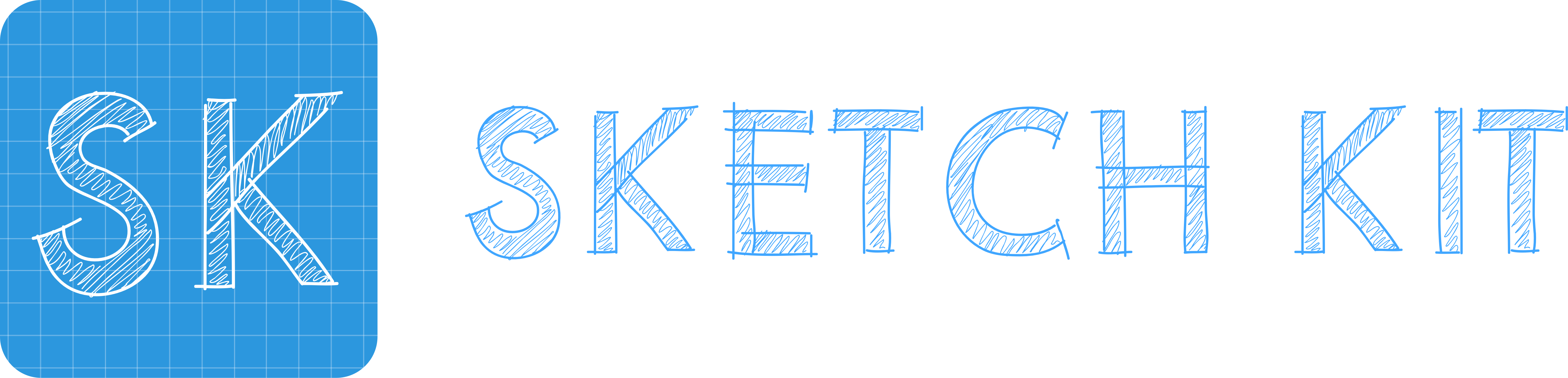
SketchKit is a lightweight, powerful and pure-Swift auto layout library, you can set up your constraints with a simple and intuitive code without any stringly typing.
In short, it allows you to replace this:
newView.translatesAutoresizingMaskIntoConstraints = false
addConstraint(NSLayoutConstraint(
item: newView,
attribute: NSLayoutConstraint.Attribute.centerX,
relatedBy: NSLayoutConstraint.Relation.equal,
toItem: view,
attribute: NSLayoutConstraint.Attribute.centerX,
multiplier: 1,
constant: 0))
or
newView.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
with this
// It's NOT necessary: newView.translatesAutoresizingMaskIntoConstraints = false
newView.layout.applyConstraint { view in
view.centerXAnchor(equalTo: self.view.centerXAnchor)
view.centerYAnchor(equalTo: self.view.centerYAnchor)
}
Requirements
- iOS 9.0+ / tvOS 9.0+
- Swift 3.2+
Installation
CocoaPods
To integrate SketchKit into your Xcode project using CocoaPods, specify it in your Podfile
:
target '<Your Target Name>' do
pod 'SketchKit'
end
Then, run the following command:
$ pod install
Swift Package Manager (SPM)
To add SketchKit
as a dependency, you have to add it to the dependencies
of your Package.swift
file and refer to that dependency in your target
.
import PackageDescription
let package = Package(
name: "<Your Product Name>",
dependencies: [
.package(url: "https://github.com/dogo/SketchKit", .upToNextMajor(from: "1.0.0"))
],
targets: [
.target(
name: "<Your Target Name>",
dependencies: ["SketchKit"]),
]
)
After adding the dependency, you can fetch the library with:
$ swift package resolve
Carthage
github "dogo/SketchKit"
Usage
Quick Start
import SketchKit
final class MyViewController: UIViewController {
let myView: UIView = {
let view = UIView(frame: .zero)
view.color = .red
return view
}()
override func viewDidLoad() {
super.viewDidLoad()
self.view.addSubview(myView)
myView.layout.applyConstraint { view in
view.topAnchor(equalTo: self.view.topAnchor)
view.leadingAnchor(equalTo: self.view.leadingAnchor)
view.bottomAnchor(equalTo: self.view.bottomAnchor)
view.trailingAnchor(equalTo: self.view.trailingAnchor)
}
}
}
Documentation
The project documentation can be found (here)
Credits
- Nicholas Babo (@NickBabo) thank you for the SketchKit logo,
License
SketchKit is released under the MIT license. See LICENSE for details.
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].