Korilakkuma / Xsound
Programming Languages
Projects that are alternatives of or similar to Xsound
XSound
Web Audio API Library for Synthesizer, Effects, Visualization, Multi-Track Recording, Audio Streaming, Visual Audio Sprite ... etc
Overview
XSound is Multifunctional Library for Web Audio API.
In concrete, XSound may be useful to implement the following features.
- Create Sound
- Play the One-Shot Audio
- Play the Audio
- Play the Media
- Streaming (by WebRTC)
- MIDI (by Web MIDI API)
- MML (Music Macro Language)
- Effectors (Compressor / Wah / Equalizer / Tremolo / Phaser / Chorus / Delay / Reverb ... etc)
- Visualization (Overview in Time Domain / Time Domain / Spectrum)
- Multi-Track Recording (Create WAVE file)
- Session (by WebSocket)
- Audio Streaming
- Visual Audio Sprite
XSound don't depend on other libraries or frameworks (For example, jQuery, React).
Supported Browsers
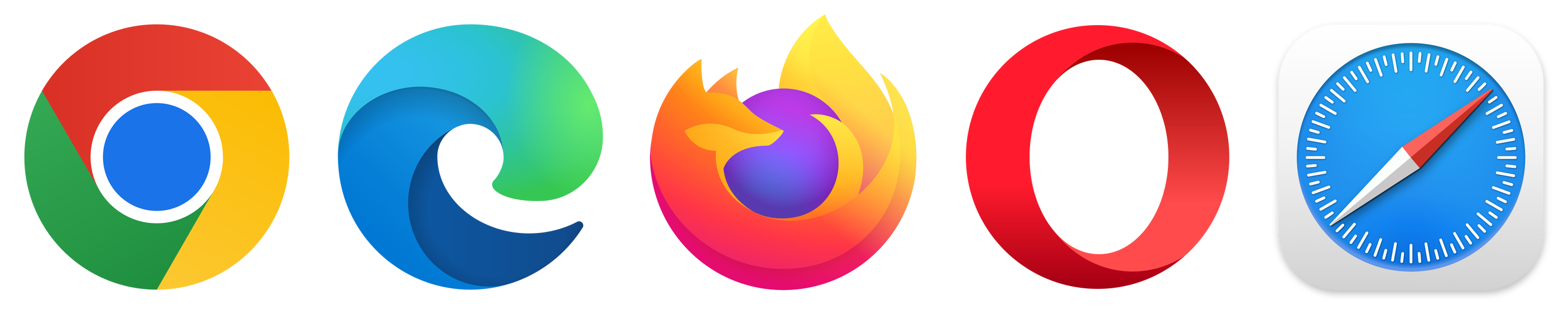
Getting Started
In the case of using as full stack (For example, use oscillator) ...
X('oscillator').setup(true).ready().start(440);
or, in the case of using as module base (For example, use chorus effector) ...
// The instance of `AudioContext`
const context = X.get();
// Create the instance of `Chorus` that is defined by XSound
// (The 2nd argument is buffer size for `ScriptProcessorNode`)
const chorus = new X.Chorus(context, 0);
const oscillator = context.createOscillator();
// The instance that is defined by XSound has connectors for input and output
oscillator.connect(chorus.INPUT);
chorus.OUTPUT.connect(context.destination);
// Set parameters for chorus
chorus.param({
time : 0.025,
depth: 0.5,
rate : 2.5,
mix : 0.5
});
// Activate
chorus.state(true);
oscillator.start(0);
XSound enable to using the following classes (Refer to API Documentation for details).
type BufferSize = 0 | 256 | 512 | 1024 | 2048 | 4096 | 8192 | 16384;
// Effectors
X.Autopanner(context: AudioContext, size: BufferSize);
X.Chorus(context: AudioContext, size: BufferSize);
X.Compressor(context: AudioContext, size: BufferSize);
X.Delay(context: AudioContext, size: BufferSize);
X.Distortion(context: AudioContext, size: BufferSize);
X.Equalizer(context: AudioContext, size: BufferSize);
X.Filter(context: AudioContext, size: BufferSize);
X.Flanger(context: AudioContext, size: BufferSize);
X.Listener(context: AudioContext, size: BufferSize);
X.Panner(context: AudioContext, size: BufferSize);
X.Phaser(context: AudioContext, size: BufferSize);
X.PitchShifter(context: AudioContext, size: BufferSize);
X.Reverb(context: AudioContext, size: BufferSize);
X.Ringmodulator(context: AudioContext, size: BufferSize);
X.Stereo(context: AudioContext, size, size: BufferSize);
X.Tremolo(context: AudioContext, size: BufferSize);
X.Wah(context: AudioContext, size: BufferSize);
X.Analyser(context: AudioContext);
X.Recorder(context: AudioContext, size: BufferSize, numberOfInputs: number, numberOfOutputs: number);
X.Session(context: AudioContext, size: BufferSize, numberOfInputs: number, numberOfOutputs, analyser: X.Analyser);
Demo
The application that uses XSound is in the following URLs.
Now, I'm creating website for Web Audio API. Please refer to the following site for understanding API Documentation.
Playground
You can view overview on YouTube.
Installation
$ npm install --save xsound
Usage
In the case of using CDN,
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/[email protected]/build/xsound.min.js"></script>
In the case of using ESModules for SSR ... etc,
import { XSound, X } from 'xsound';
Setup
Use Webpack Dev Server
$ git clone [email protected]:Korilakkuma/XSound.git
$ cd XSound
$ npm install
$ npm run dev
$ open http://localhost:8080/playground/
Use Docker
$ git clone [email protected]:Korilakkuma/XSound.git
$ cd XSound
$ npm install
$ npm run watch
$ docker-compose up -d --build
$ open http://localhost:8080/playground/
API Documentation
Pickups
-
9 libraries to kickstart your Web Audio stuff - DEV Community
-
XSound is a batteries-included library for everything audio. From basic management and loading through streaming, effects, ending with visualizations and recording, this libraries provides almost everything! It also has nice, semi-chainable API with solid documentation.
-
- 20 Useful Web Audio Javascript Libraries – Bashooka
- Extending X3D Realism with Audio Graphs, Acoustic Properties and 3D Spatial Sound
License
Released under the MIT license