xFAANG / Askql
Programming Languages
Projects that are alternatives of or similar to Askql
AskQL
AskQL is a new Turing-complete query and programming language, which allows faster and easier communication with servers.
Instead of mere data queries or simple REST questions, AskQL allows to query a server with fully functional programs which use all the data and services the server shares in a secure way.
Why and what for?
Benefits for development process:
- Next milestone after GraphQL and REST API
- New safe query language
- Send code to servers without the need to deploy
- Send executable code instead of JSONs
Benefits for programmers:
- Asynchronous by default, no more
await
keyword - cleaner code - Processes only immutable data - fewer errors
- Compiled to a clean functional code - clear logic
Prerequisites
node >=12.14
Quick Start
Installation
In your Node project run:
npm install askql
Usage
Sample index.js file:
const askql = require("askql");
(async () => {
const result = await askql.runUntyped(
{ resources: askql.askvm.resources },
askql.parse("ask { 'hello world!' }")
);
console.log(JSON.stringify(result, null, 2));
})();
Development & Contributing
Please find all important information here:
Installation from source
-
Clone the repository
git clone [email protected]:xFAANG/askql.git
-
For Linux it is advised to install autoreconf as it is used by one of the Node packages used by AskScript Playground.
For Ubuntu/Debian run:
sudo apt-get install autoconf
-
Install dependencies:
npm i
-
Build the project:
npm run build
-
Link the project to askql command:
npm link
-
Now you should be able to launch the interpreter (we use REPL for that):
askql
Code examples
You can find all the examples in __tests__
folders (e.g. 👉 AskScript tests) or in the Usage section below.
Documentation
Find AskQL documentation here.
The Documentation is divided into 4 parts:
- AskQL Overview
- AskQL Quick Guide
- AskScript - Human Friendly Language for AskScript
- AskVM - Runtime Environment for AskQL
Try It Yourself
Do not hesitate to try it out yourself! You can also find fellow AskQL devs in our Discord community.
Tools
CLI (AskScript interpreter)
Similar to python
or node
, AskScript CLI allows the user to type AskScript programs and get immediate result.
In order to run CLI:
-
Build the code:
npm run build
-
Run:
node dist/cli.js
Every input is treated as an AskScript program. For convenience, CLI expects just the body of your program, without ask{
}
.
The editor has 2 modes - a default single-line mode and a multiline mode.
In order to enter the multiline mode, please type .editor
.
At the end of your multiline input please press Ctrl+D.
$ node dist/cli.js
🦄 .editor
// Entering editor mode (^D to finish, ^C to cancel)
const a = 'Hello'
a:concat(' world')
(Ctrl+D pressed)
Result:
string ask(let('a','Hello'),call(get('concat'),get('a'),' world'))
'Hello world'
As the output CLI always prints AskCode (which would be sent to an AskVM machine if the code was executed over the network) and the result of the AskScript program.
Usage
- Write a hello world!
In AskQL we only use single quotes:
🦄 'Hello world'
string ask('Hello world')
'Hello world'
In the response you get a compiled version of the program that is sent asynchronously to the AskVM.
- There are two number types
🦄 4
int ask(4)
4
🦄 4.2
float ask(4.2)
4.2
- Let's say we have a table of philosophers and their contribution to computer science as a score:
🦄 scorePerPhilosopher
any ask(get('scorePerPhilosopher'))
{
Aristotle: 385,
Kant: 42,
Plato: 1,
Russel: 7331,
Turing: 65536,
Wittgenstein: 420
}
Now let's find out the max score with a simple query:
🦄 max(scorePerPhilosopher)
int ask(call(get('max'),get('scorePerPhilosopher')))
65536
Nice!
- Write a first query, it can be a multi-liner. First step:
.editor
second step, we write the query:
query {
philosophers
}
and here we have the answer:
any ask(query(node('philosophers',f(get('philosophers')))))
{
philosophers: [ 'Aristotle', 'Kant', 'Plato', 'Russel', 'Turing', 'Wittgenstein' ]
}
- You want to know now which philosopher had the greatest contribuition to IT, here's a one liner:
🦄 find(philosophers, fun(name) { scorePerPhilosopher:at(name):is(max(scorePerPhilosopher)) })
string ask(call(get('find'),get('philosophers'),fun(let('name',get('$0')),call(get('is'),call(get('at'),get('scorePerPhilosopher'),get('name')),call(get('max'),get('scorePerPhilosopher'))))))
'Turing'
- Exit the console!
ctrl + d
- You finished the AskScript tutorial, congratulations! 🎉
Playground
Here is the link to our AskQL playground!
Developer info - how to run Playground frontend
-
Copy .env.example to .env and set
PLAYGROUND_PORT
andPLAYGROUND_ASK_SERVER_URL
appropriately. You can also set the optionalGTM
variable to your Google Tag Manager code.or
You can also specify the variables in command line when running the Playground.
-
Compile Playground:
npm run playground:build
or
npm run build
-
Run it:
npm run playground:start
You can specify port and server URL in command line:
PLAYGROUND_PORT=1234 npm run playground:start
Additional notes
Some files in the Playground come or are inspired by https://github.com/microsoft/TypeScript-Node-Starter (MIT) and https://github.com/Coffeekraken/code-playground (MIT).
Developer info - how to run Playground backend
-
Run:
npm run build
-
Run:
node dist/playground-backend/express/demoAskScriptServer.js
If you want to specify custom port, run:
PORT=1234 node dist/playground-backend/express/demoAskScriptServer.js
instead.
FAQ
ask { <askscript> }
and eval( <javascript> )
?
What's the difference between JavaScript's eval( <javascript> )
is terrible at ensuring security. One can execute there any code on any resources available in Javascript. Moreover there is no control over time of execution or stack size limit.
On contrary, Ask's ask { <askscript> }
runs by default on a secure, sandboxed AskVM, which has a separate execution context. We have built in control mechanisms that only allow using external resources you configured. Ask programs are also run with the limits on execution time and stack size restrictions you define.
Troubleshooting
If you didn't find answers to your questions here, write on our Discord community. We will both help you with the first steps and discuss more advanced topics.
License
The code in this project is licensed under MIT license.
Core Developers
- Marcin Hagmajer (ex-Facebook)
- Łukasz Czerwiński (ex-Google)
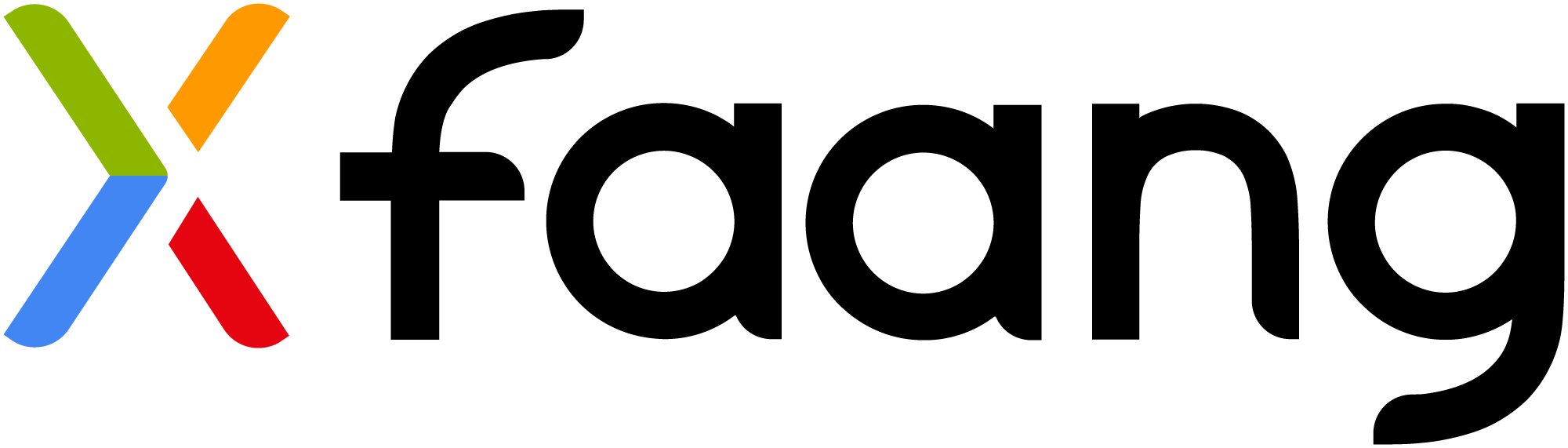