phimage / Callbackurlkit
Projects that are alternatives of or similar to Callbackurlkit
CallbackURLKit - Inter app communication
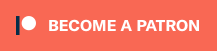
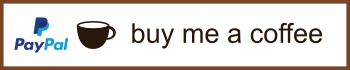
Starting to integrate URL scheme in an app,
why not be compliant with x-callback-url.
CallbackURLKit.register(action: "play") { parameters, ... in
self.player.play()
}
Want to interact with one of the numerous other applications which implement already x-callback-url, you can also use this framework.
CallbackURLKit.perform(action: "open", urlScheme: "googlechrome-x-callback",
parameters: ["url": "http://www.google.com"])
Usage
Perform action on other applications
Anywhere in your code after imported CallbackURLKit you can call
try CallbackURLKit.perform(action: "actionName", urlScheme: "applicationName",
parameters: ["key1": "value1"])
You can also use a new Manager
or the shared instance
try Manager.shared.perform(action: "actionName", urlScheme: "application-name",
parameters: ["key1": "value1"])
Declare targeted applications URL schemes in iOS9
You must whitelist any URL schemes your app wants to query in Info.plist under the LSApplicationQueriesSchemes key (an array of strings)
Create a client class
Alternatively you can create a new Client
object where you can define the targeted app URL scheme.
let client = Client(urlScheme: "application-url-scheme")
try client.perform(action:(..)
or create a new Client
class to add some utility methods which hide implementation details and allow to make some parameters check.
class GoogleChrome: Client {
init() {
super.init(urlScheme:"googlechrome-x-callback")
}
func open(url: String, ...) {
self.perform(action: "open", parameters: ["url": url], ...)
}
}
see full sample into Clients directory. (You can PR to add new Client
classes)
You can check that an application respond to the URL scheme using client.appInstalled
Use callback closure
Callbacks allow you to receive data from the target applications, but before doing this you need to add x-callback-url support to your app.
Then you can specify one of the 3 x-callbacks: success, failure and cancel.
try client.perform(action: "actionName",
onSuccess: { parameters in
},
onFailure: { error in
},
onCancel: {
}
)
Add x-callback-url support to your app
Register your URL scheme
Apps can declare any custom URL schemes they support. Use the URL Types section of the Info tab to specify the custom URL schemes that your app handles.
Or register your URL Scheme directly into Info.plist file:
- Add URL Types, then within Item 0
- Set Document Role to Editor.
- URL Identifier to your app bundle ID.
- Create a URL Schemes field and set Item 0 to the URL Scheme you want to use.
Apple documentation: Using URL Schemes to Communicate with Apps.
Setup framework with URL scheme
Then you must set the scheme to this framework manager
let manager = Manager.shared
manager.callbackURLScheme = "my-application-scheme"
// or get the first from Info.plist using utility method
manager.callbackURLScheme = Manager.urlSchemes?.first
Handle incoming URLs
And finally to handle incoming URLs, your application delegate should implement the following methods below.
On iOS
func application(_ app: UIApplication, open url: URL, options: [UIApplicationOpenURLOptionsKey : Any] = [:]) -> Bool {
manager.handleOpen(url: url)
return true
}
On OSX if you have no other need with URL events you can let manager do all the job by calling into applicationDidFinishLaunching
the method Manager.instance.registerToURLEvent()
Add new action
The client application will interact with your application using the following URL Structure.
[url-scheme]://x-callback-url/[action]?[x-callback parameters]&[action parameters]
An action is defined by its name (the url path), and optional action parameters
.
manager["myActionName"] = { parameters, success, failure, cancel in
// action code, using or not the parameters
...
// notify the client app with one of the callback
success(nil) // or failure(exception)
}
You can also register an action on shared Manager
instance using
CallbackURLKit.register(action: "myActionName") { parameters, success, failure, cancel in
}
Installation
Using CocoaPods
CocoaPods is a centralized dependency manager for Objective-C and Swift. Go here to learn more.
-
Add the project to your Podfile.
use_frameworks! pod 'CallbackURLKit'
-
Run
pod install
and open the.xcworkspace
file to launch Xcode.
Clients
To get an already implemented Clients use one of the subspec
pod 'CallbackURLKit/GoogleChrome'
pod 'CallbackURLKit/Ulysses' // https://ulyssesapp.com