xyproto / Onthefly
Licence: mit
🔗 Generate TinySVG, HTML and CSS on the fly
Stars: ✭ 37
Programming Languages
go
31211 projects - #10 most used programming language
Projects that are alternatives of or similar to Onthefly
Svgdom
Straightforward DOM implementation to make SVG.js run headless on Node.js
Stars: ✭ 154 (+316.22%)
Mutual labels: xml, svg, dom
Macsvg
macSVG - An open-source macOS app for designing HTML5 SVG (Scalable Vector Graphics) art and animation with a WebKit web view ➤➤➤
Stars: ✭ 789 (+2032.43%)
Mutual labels: xml, svg
Html To Image
✂️ Generates an image from a DOM node using HTML5 canvas and SVG.
Stars: ✭ 595 (+1508.11%)
Mutual labels: svg, dom
React Planner
✏️ A React Component for plans design. Draw a 2D floorplan and navigate it in 3D mode.
Stars: ✭ 846 (+2186.49%)
Mutual labels: threejs, svg
Dompurify
DOMPurify - a DOM-only, super-fast, uber-tolerant XSS sanitizer for HTML, MathML and SVG. DOMPurify works with a secure default, but offers a lot of configurability and hooks. Demo:
Stars: ✭ 8,177 (+22000%)
Mutual labels: svg, dom
Svg
Fork of the ms svg library (http://svg.codeplex.com/)
Stars: ✭ 676 (+1727.03%)
Mutual labels: svg, dom
React App
Create React App with server-side code support
Stars: ✭ 614 (+1559.46%)
Mutual labels: ssr, server-side-rendering
React Lazy Load Image Component
React Component to lazy load images and components using a HOC to track window scroll position.
Stars: ✭ 755 (+1940.54%)
Mutual labels: ssr, server-side-rendering
Loadable Components
The recommended Code Splitting library for React ✂️✨
Stars: ✭ 6,194 (+16640.54%)
Mutual labels: ssr, server-side-rendering
Nn Svg
Publication-ready NN-architecture schematics.
Stars: ✭ 805 (+2075.68%)
Mutual labels: threejs, svg
React Imported Component
✂️📦Bundler-independent solution for SSR-friendly code-splitting
Stars: ✭ 525 (+1318.92%)
Mutual labels: ssr, server-side-rendering
React Svg
🎨 A React component that injects SVG into the DOM.
Stars: ✭ 536 (+1348.65%)
Mutual labels: svg, dom
Jeelizweboji
JavaScript/WebGL real-time face tracking and expression detection library. Build your own emoticons animated in real time in the browser! SVG and THREE.js integration demos are provided.
Stars: ✭ 835 (+2156.76%)
Mutual labels: threejs, svg
On The Fly
- Package for generating SVG (TinySVG) on the fly.
- Can also be used for generating HTML, XML or CSS (or templates).
- HTML and CSS can be generated together, but be presented as two seperate (but linked) files.
- Could be used to set up a diskless webserver that generates all the content on the fly (something similar could also be achieved by using templates that are not stored on disk).
New/experimental features:
- Generating WebGL graphics with Three.JS on the fly.
- Generating AngularJS applications on the fly.
Online API Documentation
Generate HTML, CSS and SVG in one go
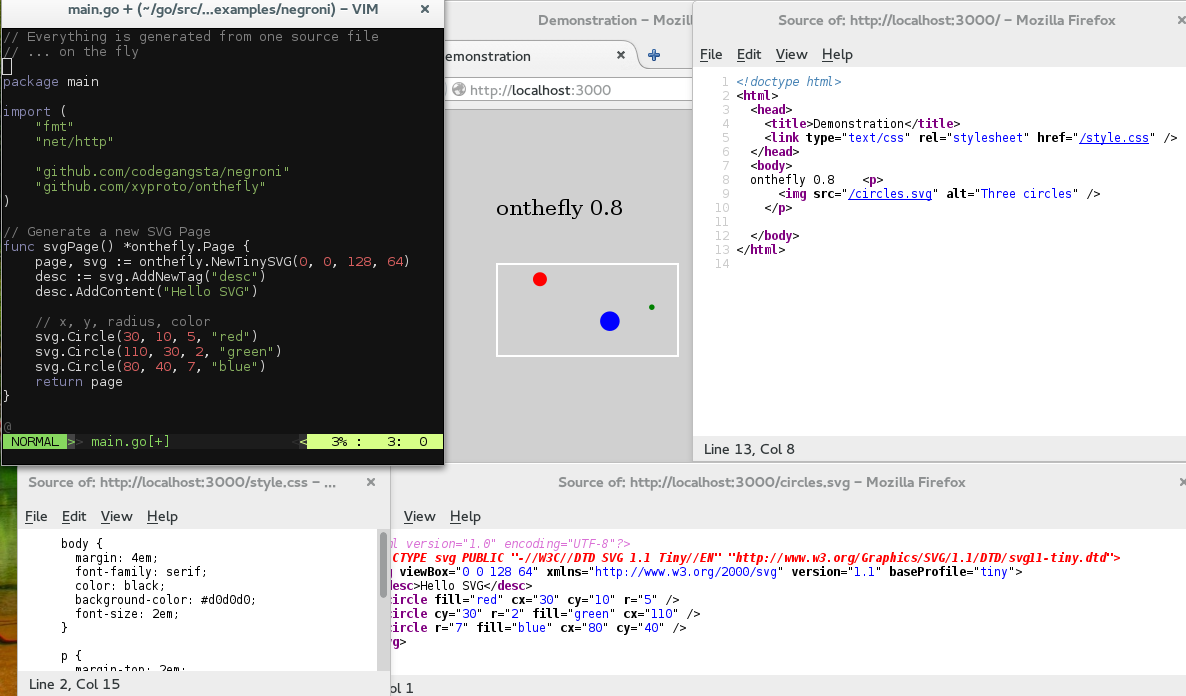
three.js
Create hardware accelerated 3D-graphics with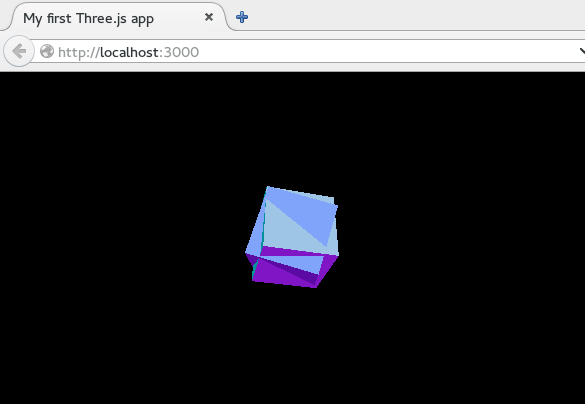
AngularJS
Experiment with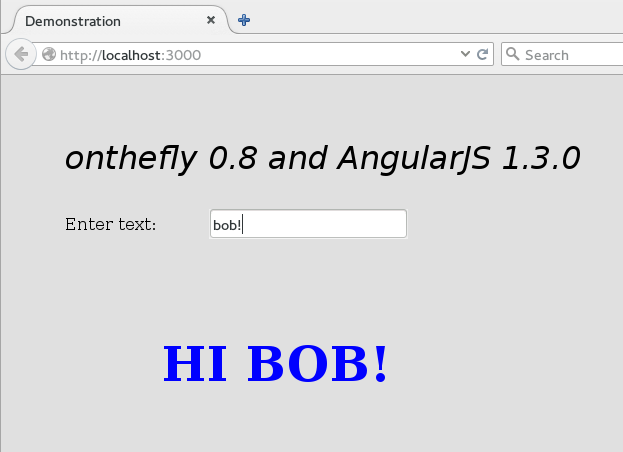
Negroni
Example forpackage main
import (
"fmt"
"net/http"
"github.com/urfave/negroni"
"github.com/xyproto/onthefly"
)
// Generate a new SVG Page
func svgPage() *onthefly.Page {
page, svg := onthefly.NewTinySVG(0, 0, 128, 64)
desc := svg.AddNewTag("desc")
desc.AddContent("Hello SVG")
// x, y, radius, color
svg.Circle(30, 10, 5, "red")
svg.Circle(110, 30, 2, "green")
svg.Circle(80, 40, 7, "blue")
// x, y, font size, font family, text and color
svg.Text(3, 60, 6, "Courier", "There will be cake", "#394851")
return page
}
// Generate a new onthefly Page (HTML5 and CSS combined)
func indexPage(svgurl string) *onthefly.Page {
// Create a new HTML5 page, with CSS included
page := onthefly.NewHTML5Page("Demonstration")
// Add some text
page.AddContent(fmt.Sprintf("onthefly %.1f", onthefly.Version))
// Change the margin (em is default)
page.SetMargin(4)
// Change the font family
page.SetFontFamily("serif") // or: sans-serif
// Change the color scheme
page.SetColor("black", "#d0d0d0")
// Include the generated SVG image on the page
body, err := page.GetTag("body")
if err == nil {
// CSS attributes for the body tag
body.AddStyle("font-size", "2em")
body.AddStyle("font-family", "sans-serif")
// Paragraph
p := body.AddNewTag("p")
// CSS style
p.AddStyle("margin-top", "2em")
// Image tag
img := p.AddNewTag("img")
// HTML attributes
img.AddAttrib("src", svgurl)
img.AddAttrib("alt", "Three circles")
// CSS style
img.AddStyle("width", "60%")
img.AddStyle("border", "4px solid white")
}
return page
}
// Set up the paths and handlers then start serving.
func main() {
fmt.Println("onthefly ", onthefly.Version)
// Create a Negroni instance and a ServeMux instance
n := negroni.Classic()
mux := http.NewServeMux()
// Publish the generated SVG as "/circles.svg"
svgurl := "/circles.svg"
mux.HandleFunc(svgurl, func(w http.ResponseWriter, req *http.Request) {
w.Header().Add("Content-Type", "image/svg+xml")
fmt.Fprint(w, svgPage())
})
// Generate a Page that includes the svg image
page := indexPage(svgurl)
// Publish the generated Page in a way that connects the HTML and CSS
page.Publish(mux, "/", "/style.css", false)
// Handler goes last
n.UseHandler(mux)
// Listen for requests at port 3000
n.Run(":3000")
}
web.go
Example forpackage main
import (
"fmt"
"github.com/hoisie/web"
"github.com/xyproto/onthefly"
"github.com/xyproto/webhandle"
)
// Generate a new SVG Page
func svgPage() *onthefly.Page {
page, svg := onthefly.NewTinySVG(0, 0, 128, 64)
desc := svg.AddNewTag("desc")
desc.AddContent("Hello SVG")
// x, y, radius, color
svg.Circle(30, 10, 5, "red")
svg.Circle(110, 30, 2, "green")
svg.Circle(80, 40, 7, "blue")
// x, y, font size, font family, text and color
svg.Text(3, 60, 6, "Courier", "There will be cake", "#394851")
return page
}
// Generator for a handle that returns the generated SVG content.
// Also sets the content type.
func svgHandlerGenerator() func(ctx *web.Context) string {
page := svgPage()
return func(ctx *web.Context) string {
ctx.ContentType("image/svg+xml")
return page.String()
}
}
// Generate a new onthefly Page (HTML5 and CSS)
func indexPage(cssurl string) *onthefly.Page {
page := onthefly.NewHTML5Page("Demonstration")
// Link the page to the css file generated from this page
page.LinkToCSS(cssurl)
// Add some text
page.AddContent(fmt.Sprintf("onthefly %.1f", onthefly.Version))
// Change the margin (em is default)
page.SetMargin(7)
// Change the font family
// Change the color scheme
page.SetColor("#f02020", "#101010")
// Include the generated SVG image on the page
body, err := page.GetTag("body")
if err == nil {
// CSS attributes for the body tag
body.AddStyle("font-size", "2em")
body.AddStyle("font-family", "sans-serif")
// Paragraph
p := body.AddNewTag("p")
// CSS style
p.AddStyle("margin-top", "2em")
// Image tag
img := p.AddNewTag("img")
// HTML attributes
img.AddAttrib("src", "/circles.svg")
img.AddAttrib("alt", "Three circles")
// CSS style
img.AddStyle("width", "60%")
}
return page
}
func main() {
fmt.Println("onthefly ", onthefly.Version)
// Connect the url for the HTML and CSS with the HTML and CSS generated from indexPage
webhandle.PublishPage("/", "/style.css", indexPage)
// Connect /circles.svg with the generated handle
web.Get("/circles.svg", svgHandlerGenerator())
// Listen for requests at port 3000
web.Run(":3000")
}
net/http
Example for just package main
import (
"fmt"
"log"
"net/http"
"time"
"github.com/xyproto/onthefly"
)
// Generate a new SVG Page
func svgPage() *onthefly.Page {
page, svg := onthefly.NewTinySVG(0, 0, 128, 64)
desc := svg.AddNewTag("desc")
desc.AddContent("Hello SVG")
// x, y, radius, color
svg.Circle(30, 10, 5, "red")
svg.Circle(110, 30, 2, "green")
svg.Circle(80, 40, 7, "blue")
// x, y, font size, font family, text and color
svg.Text(3, 60, 6, "Courier", "There will be cake", "#394851")
return page
}
// Generate a new onthefly Page (HTML5 and CSS combined)
func indexPage(svgurl string) *onthefly.Page {
// Create a new HTML5 page, with CSS included
page := onthefly.NewHTML5Page("Demonstration")
// Add some text
page.AddContent(fmt.Sprintf("onthefly %.1f", onthefly.Version))
// Change the margin (em is default)
page.SetMargin(4)
// Change the font family
page.SetFontFamily("serif") // or: sans-serif
// Change the color scheme
page.SetColor("black", "#d0d0d0")
// Include the generated SVG image on the page
body, err := page.GetTag("body")
if err == nil {
// CSS attributes for the body tag
body.AddStyle("font-size", "2em")
body.AddStyle("font-family", "sans-serif")
// Paragraph
p := body.AddNewTag("p")
// CSS style
p.AddStyle("margin-top", "2em")
// Image tag
img := p.AddNewTag("img")
// HTML attributes
img.AddAttrib("src", svgurl)
img.AddAttrib("alt", "Three circles")
// CSS style
img.AddStyle("width", "60%")
img.AddStyle("border", "4px solid white")
}
return page
}
// Set up the paths and handlers then start serving.
func main() {
fmt.Println("onthefly ", onthefly.Version)
// Create a mux
mux := http.NewServeMux()
// Publish the generated SVG as "/circles.svg"
svgurl := "/circles.svg"
mux.HandleFunc(svgurl, func(w http.ResponseWriter, req *http.Request) {
w.Header().Add("Content-Type", "image/svg+xml")
fmt.Fprint(w, svgPage())
})
// Generate a Page that includes the svg image
page := indexPage(svgurl)
// Publish the generated Page in a way that connects the HTML and CSS
page.Publish(mux, "/", "/style.css", false)
// Configure the HTTP server and permissionHandler struct
s := &http.Server{
Addr: ":3000",
Handler: mux,
ReadTimeout: 10 * time.Second,
WriteTimeout: 10 * time.Second,
MaxHeaderBytes: 1 << 20,
}
log.Println("Listening for requests on port 3000")
// Start listening
log.Fatal(s.ListenAndServe())
}
Additional screenshots
Screenshots from version 0.8:
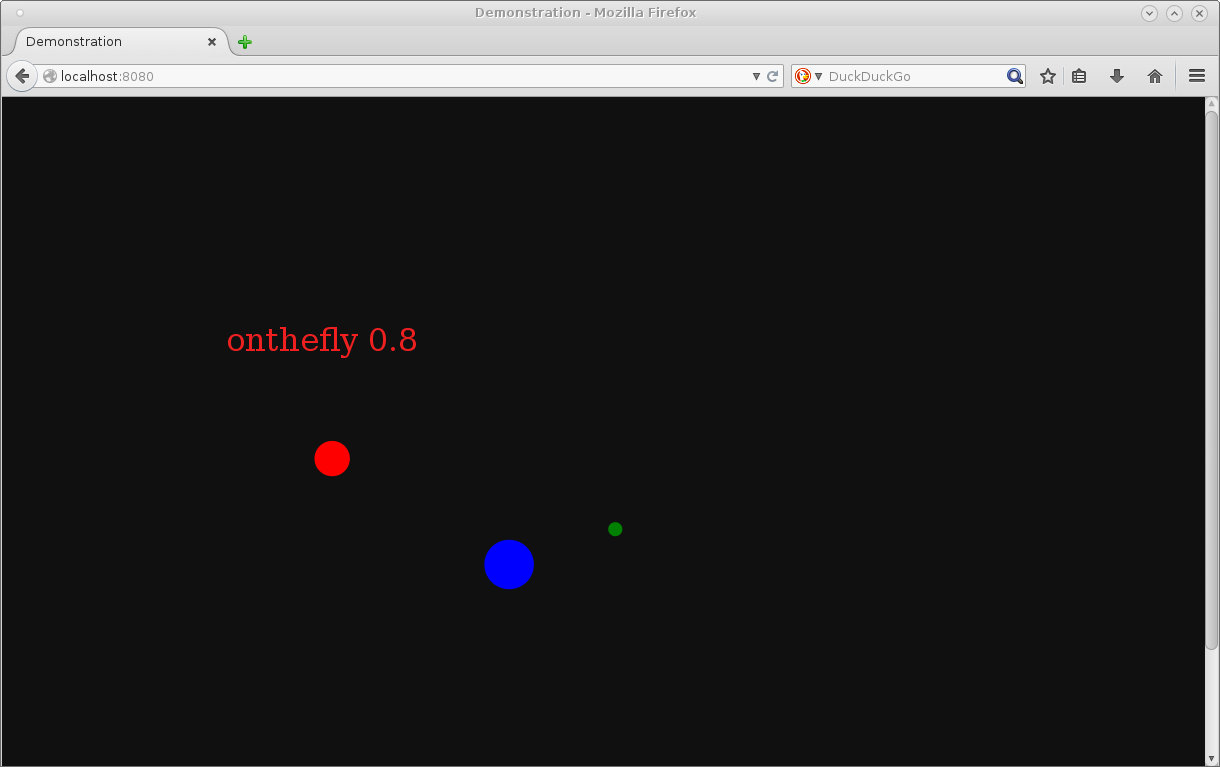
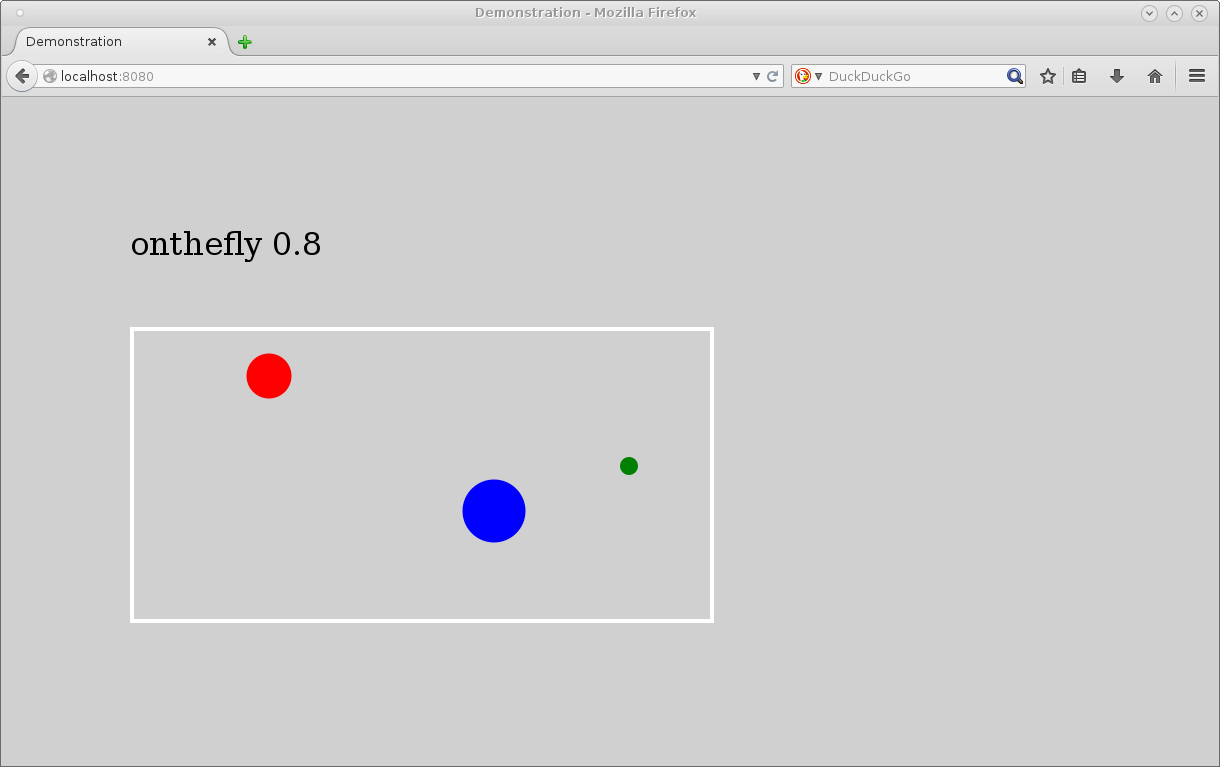
TODO
- Create a version 2 that is more focused on performance and has more consistent API function names.
Version, license and author
- Version: 0.9
- Alexander F Rødseth <[email protected]>
- License: MIT
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].