BigJk / Ramen
Licence: apache-2.0
A simple console emulator for ascii games written in go
Stars: ✭ 35
Projects that are alternatives of or similar to Ramen
Sadconsole
A .NET ascii/ansi console engine written in C# for MonoGame and XNA. Create your own text roguelike (or other) games!
Stars: ✭ 853 (+2337.14%)
Mutual labels: game-engine, roguelike, ascii
cavernos
Retro fantasy terminal for building DOS-era ASCII games, powered by WebAssembly
Stars: ✭ 32 (-8.57%)
Mutual labels: ascii, roguelike
Allure
Allure of the Stars is a near-future Sci-Fi roguelike and tactical squad combat game written in Haskell; please offer feedback, e.g., after trying out the web frontend version at
Stars: ✭ 149 (+325.71%)
Mutual labels: roguelike, ascii
Axes-Armour-Ale
A fantasy, ASCII dungeon crawler for Windows, Linux & OSX
Stars: ✭ 22 (-37.14%)
Mutual labels: ascii, roguelike
Terminal dungeon
Doom-like raycasting engine that renders to ascii for playing in terminal
Stars: ✭ 179 (+411.43%)
Mutual labels: game-engine, ascii
Game
⚔️ An online JavaScript 2D Medieval RPG.
Stars: ✭ 388 (+1008.57%)
Mutual labels: game-engine, roguelike
Lambdahack
Haskell game engine library for roguelike dungeon crawlers; please offer feedback, e.g., after trying out the sample game with the web frontend at
Stars: ✭ 439 (+1154.29%)
Mutual labels: roguelike, ascii
Amethyst
Data-oriented and data-driven game engine written in Rust
Stars: ✭ 7,682 (+21848.57%)
Mutual labels: game-engine
Dotfeather
A closs-platform generic gameengine built on C#/.NET Standard 2.1
Stars: ✭ 28 (-20%)
Mutual labels: game-engine
Dodgem
A Simple Multiplayer Game, built with Mage Game Engine.
Stars: ✭ 12 (-65.71%)
Mutual labels: game-engine
Unnethackplus
A variant of UnNetHack, development stopped
Stars: ✭ 13 (-62.86%)
Mutual labels: roguelike
Spartanengine
Game engine with an emphasis on architectual quality and performance
Stars: ✭ 869 (+2382.86%)
Mutual labels: game-engine
Video To Ascii
It is a simple python package to play videos in the terminal using characters as pixels
Stars: ✭ 960 (+2642.86%)
Mutual labels: ascii
Rogue Craft Sp
Rogue Craft is an ncurses based roguelike/sandbox/RPG game
Stars: ✭ 12 (-65.71%)
Mutual labels: roguelike
Monogame
One framework for creating powerful cross-platform games.
Stars: ✭ 8,014 (+22797.14%)
Mutual labels: game-engine
Sparky
Cross-Platform High Performance 2D/3D game engine for people like me who like to write code.
Stars: ✭ 959 (+2640%)
Mutual labels: game-engine
Ktx
LibKTX: Kotlin extensions for LibGDX games and applications
Stars: ✭ 913 (+2508.57%)
Mutual labels: game-engine

ramen is a simple console emulator written in go that can be used to create various ascii / text (roguelike) games. It's based on the great ebiten library and inspired by libraries like libtcod.
Warning: This is still a early version so api and features are not fixed yet. Bugs will happen!
Features
- PNG Fonts with more than 256 chars possible
- Fonts can contain chars and colored tiles
- Create sub-consoles to organize rendering
- Component based ui system
- Inlined color definitions in strings
- Pre-build components ready to use
- TextBox
- Button
- REXPaint file parsing
- Everything ebiten can do
- Input: Mouse, Keyboard, Gamepads, Touches
- Audio: MP3, Ogg/Vorbis, WAV, PCM
- ...
Get Started
go get github.com/BigJk/ramen/...
Example
package main
import (
"github.com/BigJk/ramen/console"
"github.com/BigJk/ramen/consolecolor"
"github.com/BigJk/ramen/font"
"github.com/BigJk/ramen/t"
"github.com/hajimehoshi/ebiten"
"fmt"
)
func main() {
// load a font you like
font, err := font.New("../../fonts/terminus-11x11.png", 11, 11)
if err != nil {
panic(err)
}
// create a 50x30 cells console with the title 'ramen example'
con, err := console.New(50, 30, font, "ramen example")
if err != nil {
panic(err)
}
// set a tick hook. This function will be executed
// each tick (60 ticks per second by default) even
// when the fps is lower than 60fps. This is a good
// place for your game logic.
//
// The timeDelta parameter is the elapsed time in seconds
// since the last tick.
con.SetTickHook(func(timeElapsed float64) error {
// your game logic
return nil
})
// set a pre-render hook. This function will be executed
// each frame before the drawing happens. This is a good
// place to draw onto the console, because it only executes
// if a draw is really about to happen.
//
// The timeDelta parameter is the elapsed time in seconds
// since the last frame.
con.SetPreRenderHook(func(screen *ebiten.Image, timeDelta float64) error {
con.ClearAll(t.Background(consolecolor.New(50, 50, 50)))
con.Print(2, 2, "Hello!\nTEST\n Line 3", t.Foreground(consolecolor.New(0, 255, 0)), t.Background(consolecolor.New(255, 0, 0)))
con.Print(2, 7, fmt.Sprintf("TPS: %0.2f\nFPS: %0.2f\nElapsed: %0.4f", ebiten.CurrentFPS(), ebiten.CurrentFPS(), timeDelta))
return nil
})
// start the console with a scaling of 1
con.Start(1)
}
Inlined Color Definitions
The console.Print function supports parsing of inlined color definitions that can change the forground and background color of parts of the string.
[[f:#ff0000]]red foreground\n[[f:#ffffff|b:#000000]]white foreground and black background\n[[b:#00ff00]]green background

Screenshots
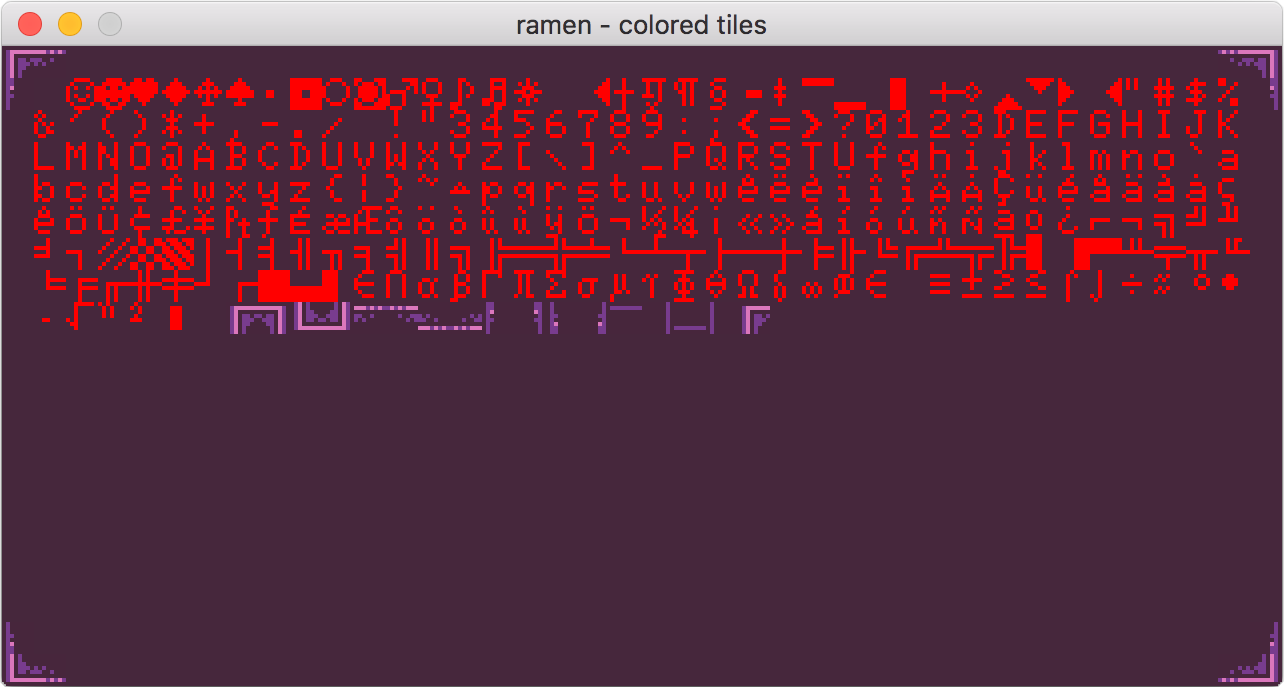
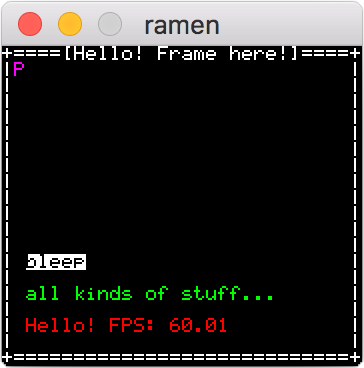
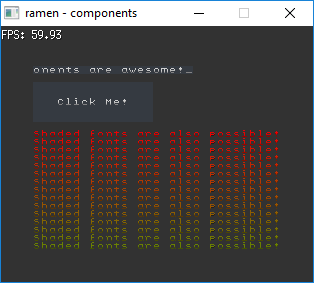
Note that the project description data, including the texts, logos, images, and/or trademarks,
for each open source project belongs to its rightful owner.
If you wish to add or remove any projects, please contact us at [email protected].