giladreich / Qtdirect3d
Programming Languages
Projects that are alternatives of or similar to Qtdirect3d
Qt Direct3D / DirectX Widgets
This project contains Direct3D widgets that can be used within the Qt Framework for DirectX 9, 10, 11 and 12.
There are also Direct3D widgets included that support Dear ImGui.
Contents
Getting Started
Clone the repository:
git clone --recursive https://github.com/giladreich/QtDirect3D.git
The main directories are source
and examples
:
Directory | Description |
---|---|
source | Qt custom widgets that you can copy to your projects depending on the Direct3D version you use. Note that under each Direct3D version, there is also the same widget with ImGui integration. |
examples | Showing how to integrate and interact with the widget. |
Building Examples
Using the Widget
In your Qt Widgets Application project, do the following steps:
- Copy the widget into your project and add it as an existing item.
- Open
MainWindow.ui
with QtDesigner and promote thecenteralWidget
to the widget you copied. - Rename
centeralWidget
toview
. - Compile to let Qt's uic & moc compiler to do their magic and have proper IntelliSense.
At this point you should have a basic setup to get started and interact with the widget.
Open MainWindow.h
file and create the following Qt slots:
public slots:
void init(bool success);
void tick();
void render();
void renderUI();
Slot | Remarks |
---|---|
bool init(bool success) | Additional initialization step. success will be true if the widget successfully initialized. |
void tick() | Update the scene, e.g. change vertices positions. |
void render() | Present the scene. |
void renderUI() | Present and manage ImGui windows. |
Open MainWindow.cpp
and connect the Widget's signals to the previously created slots:
connect(ui->view, &QDirect3DXXWidget::deviceInitialized, this, &MainWindow::init);
connect(ui->view, &QDirect3DXXWidget::ticked, this, &MainWindow::tick);
connect(ui->view, &QDirect3DXXWidget::rendered, this, &MainWindow::render);
connect(ui->view, &QDirect3DXXWidget::renderedUI, this, &MainWindow::renderUI);
As a final step, add the following code to the end of the MainWindow::init
function:
QTimer::singleShot(500, this, [&] { ui->view->run(); });
A short delay of 500 milliseconds before the frames are executed ensures that all Qt's internal signals and slots have finished processing.
Handling Close Event
It is necessary to override the closeEvent in order to properly release any used resources:
void MainWindow::closeEvent(QCloseEvent * event)
{
event->ignore();
ui->view->release();
m_bWindowClosing = true;
QTime dieTime = QTime::currentTime().addMSecs(500);
while (QTime::currentTime() < dieTime)
QCoreApplication::processEvents(QEventLoop::AllEvents, 100);
event->accept();
}
Calling the function event->ignore()
at the beginning will postpone the closeEvent
allowing for calling the widget's function release
. Lastly, giving the application a short delay of 500 milliseconds before accepting the closeEvent
.
Preview
Using the widget with imgui and creating a basic scene:
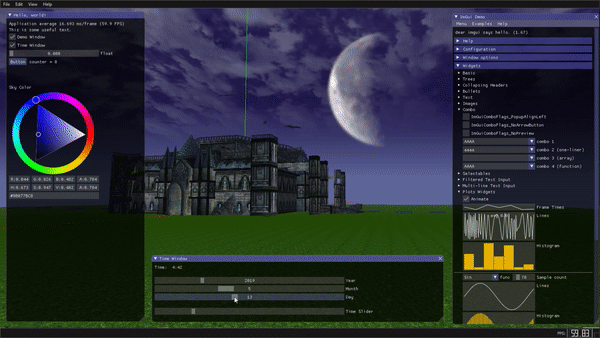
Game Engine editor using the widget (YouTube playlist):



Motivation
I've been working with both MFC GUI applications as well as Qt in various projects.
Whilst rewriting some of the MFC applications to use Qt, I noticed that Qt only provides QOpenGLWidget, but no QDirect3DWidget. I therefore began to research different ways to accomplish this.
I realized Qt's internal rendering engine needed to be disabled in order to render into the widget's surface without the internal engine's interference. Once this was achieved, it was thrilling to use the power of Qt, whilst also using Direct3D as the graphics API.
Contributing
Pull-Requests are greatly appreciated should you like to contribute to the project.
Same goes for opening issues; if you have any suggestions, feedback or you found any bugs, please do not hesitate to open an issue.
Authors
- Gilad Reich - Initial work - giladreich
See also the list of contributors who participated in this project.
License
This project is licensed under the MIT License - see the license for more details.